By: Joydip Kanjilal | Comments (1) | Related: > Entity Framework
Problem
Entity Framework Core (EF Core) is an open source, lightweight and extensible version of Entity Framework that runs on top of the .NET Core runtime and can be used to model your entities much the same way you do with Entity Framework.
Eager Loading may be defined as the process of loading the related entities of an entity as part of the initial query. This helps because you wouldn't have to load the related entities using a separate query. In other words, in eager loading the related data is loaded from the underlying database as part of the initial query. Like Entity Framework 6, Entity Framework Core supports eager loading of entities. This saves you from having to execute additional queries for the related entities.
Entity Framework Core provides support for Eager Loading using the Include() extension method. This article presents a discussion on how we can work with Eager Loading in Entity Framework Core.
Solution
In this section we’ll explore how we can work with Eager Loading in Entity Framework Core.
Getting started
In this example, we’ll use Visual Studio 2019. If you don’t have a copy of Visual Studio 2019 installed in your system, you can download a copy from here.
Follow the steps outlined below to create a new console application project in Visual Studio.
- Open Visual Studio 2019
- On the File menu, click on New > Project
- In the "Create a new project" dialog, select "Console App (.NET Core)"
- Click Next
- Now specify the name and location of the project
- Click Create
A new console application project will get created. We'll use this project in the subsequent sections of this article to examine EagerLoading.
We’ll be using the AdventureWorks database in this article to avoid having to create database tables. You can download a copy of the AdventureWorks database from here.
Once you have downloaded the AdventureWorks database, follow the steps outlined below to restore it in your system.
- Launch SQL Server Management Studio
- Click on Databases > Restore database...
- Next, select the database you would like to be restored
- Lastly, specify the name and path of the destination database
- Click OK to complete the process
Once the AdventureWorks database has been restored in your system, you’re all set to use it.
Installing the necessary Entity Framework Core packages
To be able to use Entity Framework Core, you need the necessary packages to be installed onto your project. To do this, run the following commands at the Package Manager Console.
Install-Package Microsoft.EntityFrameworkCore Install-Package Microsoft.EntityFrameworkCore.Tools Install-Package Microsoft.EntityFrameworkCore.SqlServer
Scaffolding the database with Entity Framework Core
To create the DbContext and the Models, we’ll take advantage of the Scaffold-DbContext command. Note that the Scaffold-DbContext command can be used to scaffold a DbContext and its related entity classes for a specified database. Execute the following command at the Package Manager Console to scaffold the AdventureWorks database.
Scaffold-DbContext "Server=JOYDIP;Database=AdventureWorks2017;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -o Model
Once the above command has been executed successfully, a solution folder named Model will be created and all the entity classes generated will be stored inside this folder.
Loading Related Entities in Entity Framework Core
There are three ways you can load related data in EF Core. These include the following:
- Eager Loading - Related entities are loaded as part of the initial query
- Explicit Loading - Related entities are loaded explicitly, not as part of the initial query, but at a later point of time
- Lazy Loading - Related entities are loaded when the navigation property is accessed
Working with Eager Loading in Entity Framework Core
Entity Framework Core enables you to take advantage of the Include () extension method to load related entities. In this section we’ll examine how we can work with Eager Loading in EF Core. Here’s how you can take advantage of LINQ query syntax to load related data in EF Core.
using (var context = new AdventureWorks2017Context()) { var data = (from s in context.Customer.Include("SalesOrderHeader") where s.StoreId == 1856 select s).ToList(); foreach (var result in data) Console.WriteLine(result.CustomerId); Console.Read(); }
The following code snippet illustrates how Eager Loading can be used to load related data using LINQ method syntax.
static void Main(string[] args) { using (var context = new AdventureWorks2017Context()) { var data = context.Customer .Include(n => n.SalesOrderHeader) .Where(s => s.StoreId == 1856) .ToList(); foreach(var result in data) Console.WriteLine(result.CustomerId); Console.Read(); } }
Eager Loading Multiple Entities in Entity Framework Core
You can also load related entities from several relationships in one query. The following code snippet illustrates how this can be achieved.
using (var context = new AdventureWorks2017Context()) { var data = context.Employee .Include(d => d.EmployeeDepartmentHistory) .Include(p => p.EmployeePayHistory) .ToList(); foreach (var result in data) Console.WriteLine(result.JobTitle); Console.Read(); }
Loading Multiple Levels of Related Entities in Entity Framework Core
You can use the ThenInclude() method to load multiple levels of related entities. The following code snippet illustrates how this can be achieved.
var subjects = context.Subject .Include(book => book.Books) .ThenInclude(author => author.Author) .ToList();
Here's another example - this one uses the DbContext we created earlier for the AdventureWorks2017 database.
static void Main(string[] args) { using (var context = new AdventureWorks2017Context()) { var data = context.Employee .Include(j => j.JobCandidate) .ThenInclude(b => b.BusinessEntity) .ToList(); foreach (var result in data) Console.WriteLine(result.JobTitle); Console.Read(); } }
Note that you can even chain multiple calls to the ThenInclude() extension method to load data in multiple levels. The following code snippet illustrates how this can be achieved.
var subjects = context.Subjects .Include(book => book.Books) .ThenInclude(author => author.Author) .ThenInclude(contact => contact.Contact) .ToList();
Here's another example that shows how multiple calls to the ThenInclude() extension method can be chained.
var data = context.Customer .Include(d => d.SalesOrderHeader) .ThenInclude(p => p.SalesOrderDetail) .ThenInclude(t => t.SalesOrder) .ToList();
Explicitly Loading Related Entities in Entity Framework Core
You can also explicitly load the related entities. The following query first loads a subject and then loads the books related to that subject using the Load() extension method.
var subject = context.Subjects.Single(s => s.SubjectId == 1); context.Books.Where(b => b.BookId == subject.BookId).Load();
Next Steps
- EF Core enables you to load related entities via navigation properties. Eager loading helps you to load the related entities as part of the initial query. You can use a combination of the Include() and ThenInclude() extension methods in EF Core to load related entities at multiple levels as well.
- Check out all of the Entity Framework tips on MSSQLTips.com.
About the author
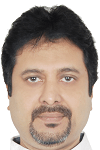
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips