By: Joydip Kanjilal | Comments | Related: > Entity Framework
Problem
Entity Framework Core (EF Core) is the flavor or Entity Framework that runs on top of the .NET Core runtime. Incidentally, Entity Framework Core 3.0 shipped on September 23, 2019. EF Core 3.0 is based on Microsoft’s flagship ORM (Entity Framework that runs on .NET Framework) and can be used to create entity data models and perform CRUD operations against the database much the same way you do with Entity Framework.
This article presents an overview of the new features and enhancements in Entity Framework Core 3.0 with code examples wherever necessary to explain the concepts covered.
Solution
In this section we’ll explore the new features and enhancements in Entity Framework Core 3.0.
Getting Started
You should have .NET Core 3.0 runtime and Visual Studio 2019 installed in your system to be able to work with Entity Framework Core and C# 8.0. If you don’t have a copy of Visual Studio 2019 installed in your system, you can download a copy from here.
Support for C# 8.0
C# 8.0 is available as part of .NET Core 3.0. Entity Framework Core 3.0 enables you to leverage some of the striking features of C# 8.0 that includes asynchronous streams, nullable reference types, etc. You can now take advantage of the IAsyncEnumerable<T> interface for working with async queries.
Here's an example that illustrates how this can be achieved.
var authors = from a in context.Authors where a.IsActive == true select a; await foreach(var v in authors.AsAsyncEnumerable()) { DisplayAuthor(v); }
The following code snippet illustrates how you can define a class that contains a nullable reference type.
public class Author { public int Id { get; set; } public string FirstName { get; set; } public string? MiddleName { get; set; } public string LastName { get; set; } }
Installing the necessary packages
Entity Framework Core 3.0 is available as a set of NuGet packages. If you would like to work with Entity Framework Core and SQL Server in your applications, you would need to add the SQL Server provider using the following command:
Install-Package Microsoft.EntityFrameworkCore.SqlServer
Note that the Entity Framework Core command-line tool dotnet ef is no longer a part of the .NET Core SDK. You should install dotnet-ef as a global tool to be able to scaffold a DbContext in Entity Framework Core 3.0.
Support for Cosmos DB
Cosmos DB is a lightweight, multi-model database service from the software giant Microsoft that is available in Azure. Entity Framework Core provides support for working with Cosmos DB in Azure. You may want to learn more on Cosmos DB from here.
You now have a Cosmos DB provider that can be used to configure Cosmos DB as the underlying database for your application.
No more Query types
The previous versions of Entity Framework Core used to work only with tables that have a primary key defined. Now, the results of a stored procedure or a view doesn't have a primary key. To solve this confusion, query types were introduced in Entity Framework Core. To define query types, you have had to use DbQuery<T> in lieu of DbSet<T>. This created a lot of confusion as well and there were complaints aplenty. With EF Core 3.0, query types have been removed. You would just be using DbSet for any data source. If the database table doesn't have a primary key defined, you can annotate such tables with .HasNoKey().
Indexed property
With Entity Framework Core 3.0, you can store data in indexed properties. The following code listing illustrates how indexed property can be used.
public class ThisIsATestEntity { public object this[string thisIsAProperty] { get { //Get accessor of the property named thisIsAProperty } set { //Set accessor of the property named thisIsAProperty } } }
Client-side Query Execution
In the earlier versions of Entity Framework Core for LINQ queries that couldn't be translated to SQL, the runtime used to download the database tables and then perform the operation in the memory. This proved to be a costly operation when you were using a where clause and your database table contained many rows.
The LINQ provider has been rearchitected and revamped to enable it to be able to translate more query patterns to SQL. From Entity Framework Core 3.0 onwards, LINQ queries are no longer evaluated on the client. Only the top-level projection will be evaluated on the client. If you have expressions that cannot be converted to a corresponding SQL, the runtime will throw an exception.
Single SQL Statement for each LINQ query
Generation of a single SQL statement per LINQ query is another feature in Entity Framework Core 3.0. This is contrary to the previous versions of EF Core where calls to the Include() method resulted with inefficient queries (N+1) queries.
Shared type entities
The shared type entity is a new feature introduced in Entity Framework Core 3.0. This feature enables you to use the instance of the same class to represent different entity types in the model. This feature helps you to eliminate the need of a third entity type when representing a many to many relationships.
The new Interception API
The new Interception API in Entity Framework Core 3.0 enables you to intercept and invoke custom code to be executed whenever low-level database operations are executed. In other words, you can take advantage of interception to execute custom code before and after the execution of database operations. Note that to work with interceptors in EF Core 3.0 you must register your interceptor using the AddInterceptor method.
Next Steps
- Entity Framework Core 3.0 is an attempt from Microsoft to unleash the potential of Entity Framework Core and fix the perceived deficiencies as evident in the previous versions of Entity Framework Core. There are a ton of new features and enhancements in Entity Framework Core 3.0 - we discussed some of them here. You can find out more on this from here.
- The latest release of Entity Framework Core is EF Core 3.1. As of this writing, EF Core 3.1 has just been released - it was released just 6 days ago. Microsoft will provide a long-term support for EF Core 3.0 and EF Core 3.1 - it should support these releases for 3 years. The next release of EF Core will be EF Core 5.0.
About the author
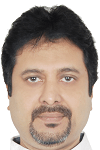
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips