By: Ray Barley | Comments | Related: > SharePoint
Problem
I'm just getting started developing some customizations for SharePoint 2010 and I'd like some help getting ramped up on how to do this using Visual Studio 2010. My initial task is to develop some web parts.
Solution
Visual Studio 2010 has built-in support for developing all sorts of SharePoint 2010 customizations. The development environment is pretty much on par with what we've become accustomed to when we use Visual Studio to develop web applications, windows forms applications, and so on. At a high level Visual Studio 2010 provides the following capabilities for developing SharePoint customizations:
- SharePoint Connections node in the Server Explorer, allowing you to examine the properties of SharePoint objects
- Project types for a variety of SharePoint customizations such as web parts, content types, workflows, and so on
- Creation of SharePoint features; a feature encapsulates functionality and can be activated and deactivated by an administrator
- Creation of the SharePoint solution package; the solution package is the primary deployment mechanism for SharePoint. It contains the features and other assets to be deployed to SharePoint.
- Deployment to a SharePoint site on your development machine for debugging and testing
- Integrated debugging
In this tip I will discuss each of the above capabilities and use the creation of a simple web part as an example to introduce SharePoint 2010 development with Visual Studio 2010.
Development Prerequisites
Let's first lay down the basics that you need in place to start developing SharePoint customizations with Visual Studio 2010. In no particular order here are the considerations for your development environment:
- You need one of the following editions of Visual Studio 2010: Professional, Premium or Ultimate. The Express versions do not have the built-in SharePoint development capabilities. The details on the specific features in each edition can be found here.
- Install everything you need for development on a single machine; this can and probably should be a virtual machine using Hyper-V, VMWare, Windows 7 or Windows 2008 Server boot from VHD, etc.
- You must use a 64 bit operating system
- Choose Windows Server 2008 (or later) as your operating system. You can also use Windows Vista or Windows 7 but these will require a bit more work to get SharePoint and its prerequisites installed and running. Take a look at Setting Up the Development Environment for SharePoint 2010 on Windows Vista, Windows 7, and Windows Server 2008 for the details; be sure to read the article thoroughly and perform every step, especially for Windows Vista or Windows 7.
- Run Visual Studio as an administrator. Right click on it in the Microsoft Visual Studio 2010 program group and select Run as Administrator from the popup menu.
Sharepoint Server Explorer
The Server Explorer includes a SharePoint Connections node as shown below. This allows you to drill down into a SharePoint site collection and browse the contents.
The information is read-only so it just provides the opportunity to examine the properties of an object. For example expand the List Templates node, expand the Tracking node, right click on Tasks, and select Properties from the popup menu. The properties for the Tasks list template are shown below:
Project Types
When you create a new SharePoint project in Visual Studio 2010, you will see the following project types available in the SharePoint 2010 Installed Templates:
As you can see most of the typical SharePoint customizations are available. Each one typically creates the appropriate artifacts that are necessary; you can just start writing code, debugging and testing. There are some SharePoint customizations that Visual Studio 2010 does not directly support such as a Custom Action; for these you have to create and / or edit the necessary files on your own.
Create a New Project
In the previous section I showed the new project dialog. In this section I will go through creating a project. To begin select the Empty SharePoint Project and fill in the dialog as shown below:
The Empty SharePoint Project is a good starting point; you can add specific SharePoint items to the project. Click OK to continue and the SharePoint Customization Wizard is displayed as shown below:
The information requested is the site collection to use for debugging and whether to deploy a sandboxed or farm solution. It is a good idea to create a site collection to use just for debugging and testing, rather than using the default site collection that gets created for you when you install and configure SharePoint. The reason is that if you get things fouled up you can delete the site collection, create a new one, and continue. The farm solution runs with full trust whereas the sandboxed solution runs with limited trust. The sandboxed solution is a great feature to use; however, there are various limitations such as restrictions on the SharePoint API, no access to the file system, and so on. For our example we can use a sandboxed solution. Click Finish to complete the creation of the new project.
The Solution Explorer and Project Properties are shown below (if necessary click View on the menu then select Solution Explorer):
The above shows us the starting point for the Empty SharePoint Project. The main points are:
- MSSharePointTipsWebParts is the name of our project
- The project properties are shown in the Properties window
- The Features node is the container for the features that will be created as we add SharePoint items to the project
- The Package node is the container for the information required to package the features and other assets into a SharePoint solution file for deployment
SharePoint Project Properties
A SharePoint project has a group of properties that we use to specify some deployment settings for our debugging environment. Right click the project in the Visual Studio Solution Explorer, select Properties from the menu, and click on SharePoint to display the settings as shown below:
The following are the main points regarding the SharePoint project properties:
- The Pre-deployment command line is executed right before deployment
- The Post-deployment command line is executed after deployment
- The Active Deployment Configuration selection allows you to specify a group of settings that control what happens when you deploy from Visual Studio
- The Default and No Activation deployment configurations are predefined; you cannot edit or delete them
- You can maintain your own deployment configurations; click New, Edit, or Delete as appropriate
Note that Auto-retract after debugging is checked; this causes Visual Studio to remove the solution features from the SharePoint server after debugging and is generally a good idea. The development process typically consists of many iterations of writing and debugging code; retracting the solution when you end your debugging session helps to keep your SharePoint site in order.
Select the Default deployment configuration and click View; the following dialog is displayed:
As you can see from the above dialog there are a number of predefined deployment and retraction steps. If necessary you can define your own deployment configuration to perform the steps that you require. Later in the Debugging section we'll see the deployment configuration steps in action.
Add a Web Part
Right click the project in the Visual Studio Solution Explorer, and click Add, New Item from the menu to display the list of SharePoint items that you can add to a project as shown below:
Select Web Part, fill in the Name, and click Add (add button is not shown above in order to fit the image). The Solution Explorer now includes the additional items as shown below:
After adding the web part, the Features folder now contains a Feature1 folder with a Feature1.feature file and there is a new folder named MSSharePointTipsSampleWebPart that has the files in it that we need to implement our web part. I'll talk about the feature in the next section; let's review the web part folder now.
Adding a web part to the project added three files to the MSSharePointTipsSampleWebPart folder:
- Elements.xml
- MSSharePointTipsSampleWebPart.vb
- MSSharePointTipsSampleWebPart.webpart
The Elements.xml file is shown below; it contains the information required to deploy the web part.
The Module node is used to put a .webpart file into the SharePoint content database. The .webpart file (described below) is added to a special document library called the Web Part Gallery. After the web part is deployed to SharePoint (described below), you can view it in the Web Part Gallery by clicking Site Actions, Site Settings, and selecting Web parts in the Galleries section as shown below:

When you navigate to the Web Part Gallery, you will notice that the URL includes _catalogs/wp which is the Url in the Module node in the Elements.xml file above. The entry for the .webpart file in the Web Part Gallery is shown below:
The entry in the Web Part Gallery reflects the Url in the File node and the Group property value from the Elements.xml file shown above.
The contents of the .webpart file are shown below:
The type node specifies the class name that implements the web part and the name of the DLL that contains the class.
The web part code is shown below (I added the three lines inside of the CreateChildControls subroutine):
Imports System Imports System.ComponentModel Imports System.Web Imports System.Web.UI Imports System.Web.UI.WebControls Imports System.Web.UI.WebControls.WebParts Imports Microsoft.SharePoint Imports Microsoft.SharePoint.WebControls <ToolboxItemAttribute(false)> _ Public Class MSSharePointTipsSampleWebPart Inherits WebPart Protected Overrides Sub CreateChildControls() Dim oLabel As New Label oLabel.Text = "My Sample Web Part" Controls.Add(oLabel) End Sub End Class
The above web part simply displays a label when added to a page.
Feature Designer
Features are individual pieces of functionality that are deployed in a SharePoint solution and can be activated or deactivated by an administrator. As SharePoint items are added to a project, a feature is created. The feature designer allows us to map the SharePoint items in the project to a feature.
Right click on the Feature1 folder in the Solution Explorer and rename it to MSSharePointTipsVS2010. Double click the MSSharePointTipsVS2010 folder to display the Feature designer as shown below:
The web part that was added is automatically included in the Items in the Feature as shown above. There are no other SharePoint items in our solution at this point. Keep in mind that when we deploy the feature, all of its items in the feature are activated or deactivated as a group. Make sure to change the Title and Description properties, as these will be what the administrator sees on the Site Collection Features page (described below). Note that the Scope is specified as Site; this means that the feature will be deployed at the site collection level, which is appropriate for a web part.
We can specify Feature Activation Dependencies which are features that are required by our feature. We can click on Manifest to review the XML as shown below:
In some instances it is necessary to manually edit the above; this is not necessary in this case. You can use the XML Editor or enter changes in the dialog.
Package Designer
The package designer allows us to specify the contents of the SharePoint solution package, which is made up of the features and other assets that are included in the project. Double click the Package folder in the Visual Studio Solution Explorer to display the package designer as shown below:
The Items in the Package shown above are included automatically as a result of adding the web part to the project. The above dialog allows us to select which items in the Visual Studio solution are included. In this case we have no other items in the Visual Studio solution available.
Click the Advanced button to specify any additional assemblies that need to be deployed with the SharePoint solution as shown below:
Click Add to specify an assembly to include in the package. You can select an assembly from the file system or the output of another project in your Visual Studio solution.
Click the Manifest button to review the details as shown below:
The manifest gets populated automatically; if necessary it can be manually edited by opening the XML Editor or making changes in the above dialog. Note in this case that the manifest specifies the SafeControl entry that is required to be added to the web.config file for a web part.
Debugging
The debugging for SharePoint projects in Visual Studio 2010 works as you would expect; you set breakpoints, launch the debugger, and your breakpoints get hit. When you create a SharePoint project and get asked the question what local site do you want to use for debugging, your answer tells Visual Studio which process to attach to for debugging. In my sample web part I set a breakpoint on the first line of code in the CreateChildControls function then start the debugger; (click Debug, Start Debugging from the menu, or press F5). At this point there's quite a bit going on. The contents of the output window are shown below when I started the debugger:
------ Build started: Project: MSSharePointTipsWebParts, Configuration: Debug Any CPU ------ MSSharePointTipsWebParts -> C:\spdemo\MSSharePointTips\MSSharePointTipsWebParts\bin\Debug\MSSharePointTipsWebParts.dll Successfully created package at: C:\spdemo\MSSharePointTips\MSSharePointTipsWebParts\bin\Debug\MSSharePointTipsWebParts.wsp ------ Deploy started: Project: MSSharePointTipsWebParts, Configuration: Debug Any CPU ------ Active Deployment Configuration: Default Run Pre-Deployment Command: Skipping deployment step because a pre-deployment command is not specified. Recycle IIS Application Pool: Skipping application pool recycle because a sandboxed solution is being deployed. Retract Solution: Skipping package retraction because no matching package on the server was found. Add Solution: Adding solution 'MSSharePointTipsWebParts.wsp'... Deploying solution 'MSSharePointTipsWebParts.wsp'... Activate Features: Activating feature 'MSSharePointTipsVS2010' ... Run Post-Deployment Command: Skipping deployment step because a post-deployment command is not specified. ========== Build: 1 succeeded or up-to-date, 0 failed, 0 skipped ========== ========== Deploy: 1 succeeded, 0 failed, 0 skipped ========== Active Deployment Configuration: Default Recycle IIS Application Pool: Skipping application pool recycle because a sandboxed solution is being deployed. Retract Solution: Deactivating feature 'MSSharePointTipsWebParts_MSSharePointTipsVS2010' ... Retracting solution 'MSSharePointTipsWebParts.wsp'... Deleting solution 'MSSharePointTipsWebParts.wsp'...
The following are the main points about what happens when you start debugging:
- The project is built
- The SharePoint solution package is created, containing everything that is to be deployed to SharePoint
- The rest of the steps are based on the Active Deployment Configuration; refer back to the SharePoint Project Properties section to view the built-in configurations and their predefined steps
One important point about the above debug output is that the lines after "Deploy: 1 succeeded, 0 failed, 0 skipped" are actually performed when the debug session is ended, showing the SharePoint solution being retracted.
Add the web part to a page and immediately the breakpoint in the web part code will be hit. The web part will render the following content on a page:
Before stopping the debugger, you can see for yourself that our feature has been installed and activated. Open your browser to the local site that you specified for debugging (e.g. http://barley-sp2010/sites/spdev in my case), click Site Actions, and Site Settings as shown below:

The Site Settings pages is displayed; click Site collection features in the Site Collection Administration section as shown below:

The list of features is displayed; you will see the feature that we deployed and it's state will be activated as shown below:
Note that the title and description shown above are what was entered earlier in the feature designer.
Next Steps
- Reference the SharePoint 2010 SDK for the many details that you'll need to successfully develop SharePoint features.
- You can find visual How Tos for creating the various SharePoint 2010 customizations with Visual Studio 2010.
About the author
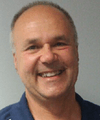
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips