By: Eli Leiba | Comments (4) | Related: > Backup
Problem
We need to create a simple piece of T-SQL code that will pre-check the free disk drive space and estimate the database backup size to determine if there is enough space to run the BACKUP DATABASE statement for the selected disk drive. This tool can be used to prevent errors concerning lack of disk space during the backup process and can save time of doing large backup operations that will eventually fail.
Solution
The solution involves creating a stored procedure in SQL Server that gets an input parameter for the disk drive letter where the database backup file will be created and an output bit parameter that its value will be:
- 1 – If the drive has enough space for the database backup file (actually 15% more than the actual size of the backup) – In this case it will be OK to continue with the backup on this drive.
- 0 – If the drive does not have enough space for the backup. In this case, don't run the backup until the disk is freed or expanded or another drive with more space is selected for the backup.
The backup estimated size is in KB and is calculated by using results taken from the sp_spaceused system stored procedure. The size of the database backup is roughly equal to the reserved space in the database as taken from the system stored procedure sp_spaceused. This procedure calculates among other values how much reserved space there is in the database. It is shown by MVP Jonathan Kehayias in the following link: http://jmkehayias.blogspot.co.il/2008/12/estimating-size-of-your-database.html. The drive free space estimation is calculated by executing the extended stored procedure xp_fixeddrives
This procedure returns a record set that contains the number of megabytes of free space for each physical drive associated with the SQL Server machine. The procedure checks that the backup estimated size (KB) multiplied by 1.15 (15% size addition) is still less than the drive free space estimation (KB) and sets the output parameter value accordingly.
SQL Server Stored Procedure to Validate Space Exists for Database Backup File
This procedure needs to be created in each user database, since it queries tables local to the database to estimate the backup size. I will be checking the Northwind database, so I will create it in that database.
-- ================================================================ -- Author: Eli Leiba -- Create date: 04-2018 -- 05-2018 -- 1. Adding database name for check -- 2. Result is in MB -- Description: Check if drive has enough space for DB backup -- ================================================================ CREATE PROCEDURE dbo.DoesDBBackupHaveSpace ( @drvLetter VARCHAR (5), @enoughSpaceForBackupFlag BIT OUTPUT ) AS BEGIN DECLARE @estimatedBackSizeMB INT DECLARE @estimatedDriveFreeSpaceMB INT DECLARE @dbCheckMessage varchar(80) SET NOCOUNT ON SET @dbCheckMessage = concat ('Checking database ', DB_NAME ()) PRINT @dbCheckMessage SELECT @estimatedBackSizeMB = round (sum (a.total_pages) * 8192 / SQUARE (1024.0), 0) FROM sys.partitions p JOIN sys.allocation_units a ON p.partition_id = a.container_id LEFT JOIN sys.internal_tables it ON p.object_id = it.object_id CREATE TABLE #freespace (drive VARCHAR (5), MBFree DECIMAL (8, 2)) INSERT INTO #freespace ( Drive, MBFree) EXEC xp_fixeddrives SELECT @estimatedDriveFreeSpaceMB = MBFree FROM #freespace WHERE drive = @drvLetter IF @estimatedBackSizeMB * 1.15 < @estimatedDriveFreeSpaceMB SET @enoughSpaceForBackupFlag = 1 ELSE SET @enoughSpaceForBackupFlag = 0 SELECT DatabaseName = db_name(), Estimated_Back_Size_MB = @estimatedBackSizeMB, Estimated_Drive_Free_Space_MB = @estimatedDriveFreeSpaceMB, EnoughSpaceForBackupFlag = @enoughSpaceForBackupFlag DROP TABLE #freespace SET NOCOUNT OFF END GO
Example Using the Stored Procedure
Let’s check my server that the C:\ drive has enough space for backing up the Northwind database.
We need to make sure we are in the database we want to check when we run the stored procedure.
USE Northwind GO DECLARE @enoughSpaceForBackupFlag bit EXEC dbo.DoesDBBackupHaveSpace 'C', @enoughSpaceForBackupFlag OUTPUT PRINT @enoughSpaceForBackupFlag IF @enoughSpaceForBackupFlag = 1 PRINT 'Continue to Backup...' ELSE PRINT 'Drive Space Problem...' GO
And the results are:
Database Name | Estimated Back Size MB | Estimated Drive Free Space MB | Enough Space For Backup Flag |
---|---|---|---|
Northwind | 9 | 49246 | 1 |
On the message tab we have this output.
Checking database Northwind 1 Continue to Backup...
This means that the C:\ drive has enough space for completing the backup of the Northwind database.
Next Steps
- You can create this simple procedure in your application database and use it as a simple T-SQL tool for pre-checking database backup operations on databases.
- The procedures were tested on SQL Server 2014, 2016 and 2017.
About the author
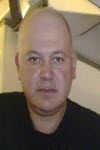
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips