By: Joydip Kanjilal | Comments | Related: > Entity Framework
Problem
Entity Framework Core (EF Core) is an open source, lightweight, cross-platform and extensible version of Microsoft’s popular ORM called Entity Framework. EF Core runs on top of the .NET Core runtime and can be used to model your entities much the same way you do with Entity Framework. This article presents a discussion on how EF Core can be used to log data in ASP.NET Core.
Solution
Logging is a cross-cutting concern that is used to capture data that can help in detecting or investigating issues in an application. EF Core provides support for logging by taking advantage of the logging mechanism in .NET Core. Interestingly, ASP.NET Core takes advantage of the same logging mechanism as well.
Getting Started
As of the writing of this article, the latest version of Visual Studio is Visual Studio 2019. We’ll use Visual Studio 2019 in this example. If you don’t have a copy of Visual Studio 2019 installed in your system, you can download a copy from here.
Creating a new ASP.NET Core project
First off, create a new console application project by following the steps outlined below:
- Open Visual Studio 2019 IDE
- On the File menu, click on the option New > Project
- In the "Create a new project" dialog, select "ASP.Net Core Web Application" and click Next
- Specify the name and location of the project in the "Configure your new project" window
- Select API as the project template and also the version of ASP.NET Core you would like to use
- Click Create to complete the process
A new ASP.NET Core Web API project will be created – we’ll take advantage of this project to demonstrate how to work with logging in EF Core in the sections that follow.
Creating the database tables
We’ll now create the required database table(s) to be used when creating the DbContext and the models. For the sake of simplicity, we’ll keep the table design simple. Create two database tables named, Author and Book using the scripts given below.
CREATE TABLE [dbo].[Author]( [AuthorId] [int] IDENTITY(1,1) NOT NULL, [FirstName] [nvarchar](50) NOT NULL, [LastName] [nvarchar](50) NOT NULL, CONSTRAINT [PK_AuthorId] PRIMARY KEY CLUSTERED ( [AuthorId] ASC )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY] ) ON [PRIMARY] GO CREATE TABLE [dbo].[Book]( [BookId] [int] IDENTITY(1,1) NOT NULL, [Title] [nvarchar](50) NOT NULL, [AuthorId] [int] NOT NULL, CONSTRAINT [PK_BookId] PRIMARY KEY CLUSTERED ( [BookId] ASC )WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY] ) ON [PRIMARY] GO ALTER TABLE [dbo].[Book] WITH NOCHECK ADD CONSTRAINT [FK_Book_Author] FOREIGN KEY([AuthorId]) REFERENCES [dbo].[Author] ([AuthorId]) GO ALTER TABLE [dbo].[Book] CHECK CONSTRAINT [FK_Book_Author] GO
Installing the necessary packages
Next, you should install a logging provider onto your project. In this post we'll be using the console logger provided by Microsoft for the sake of simplicity. To be able to use Console Logging in Entity Framework Core, you should install the Microsoft.Extensions.Logging.Console package. You can install the package from the NuGet Package Manager or from the Package Manager Console using the following command.
Install-Package Microsoft.Extensions.Logging.Console
So far so good. We’ve set up the environment for working with logging in EF Core. In the sections that follow, we’ll learn how we can log data using the console logging provider in EF Core.
Creating the DbContext
We'll take advantage of the Scaffold-DbContext command to generate the DbContext and the models. The following is the syntax of the Scaffold-DbContext command.
Scaffold-DbContext [-Connection] [-Provider] [-OutputDir] [-Context] [-Schemas>] [-Tables>] [-DataAnnotations] [-Force] [-Project] [-StartupProject] [<CommonParameters>]
If you would like to get a detailed help on how to use this command, you can execute the following command at the Package Manager Console.
get-help scaffold-dbcontext –detailed
Execute the following command at the Package Manager Console to generate the DbContext and the associated model classes.
Scaffold-DbContext "Server=JOYDIP;Database=MSSQLTips;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models
This command would create a solution folder named Models and three files inside it. These include the following:
- MSSQLTipsContext.cs
- Author.cs
- Book.cs
The Author and Book Entities
Here's how the Author and the Book entities would look like:
public partial class Author { public Author() { Book = new HashSet<Book>(); } public int AuthorId { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public virtual ICollection<Book> Book { get; set; } } public partial class Book { public int BookId { get; set; } public string Title { get; set; } public int AuthorId { get; set; } public virtual Author Author { get; set; } }
The DbContext Class
The following is the DbContext class named MSSQLTipsContext which you can use to perform CRUD operations.
public partial class MSSQLTipsContext : DbContext { public MSSQLTipsContext() { } public MSSQLTipsContext(DbContextOptions<MSSQLTipsContext> options) : base(options) { } public virtual DbSet<Author> Author { get; set; } public virtual DbSet<Book> Book { get; set; } protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { if (!optionsBuilder.IsConfigured) { optionsBuilder.UseSqlServer ("Server=JOYDIP;Database=MSSQLTips;Trusted_Connection=True;"); } } protected override void OnModelCreating(ModelBuilder modelBuilder) { modelBuilder.HasAnnotation("ProductVersion", "2.2.4-servicing-10062"); modelBuilder.Entity<Author>(entity => { entity.Property(e => e.FirstName) .IsRequired() .HasMaxLength(50); entity.Property(e => e.LastName) .IsRequired() .HasMaxLength(50); }); modelBuilder.Entity<Book>(entity => { entity.Property(e => e.Title) .IsRequired() .HasMaxLength(50); entity.HasOne(d => d.Author) .WithMany(p => p.Book) .HasForeignKey(d => d.AuthorId) .OnDelete(DeleteBehavior.ClientSetNull) .HasConstraintName("FK_Book_Author"); }); } }
Note that for security reasons, you should move the connection string out of the source code.
Creating a Logger Factory instance
Next, we need to create a logger factory. A logger factory is comprised of one or more providers. You can take advantage of the logger factory to send out logs to several destinations. Now that the console logging provider has been installed, you need to create an instance of Loggerfactory and associate it with the DbContext as shown in the code snippet given below.
public static readonly ILoggerFactory loggerFactory = new LoggerFactory(new[] { new ConsoleLoggerProvider((_, __) => true, true)});
Configuring the DbContext to use the Logger Factory
Once the logger factory has been created, you can associate the logger factory instance with the DbContext in the OnConfiguring() method as shown in the code snippet given below.
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) { if (!optionsBuilder.IsConfigured) { optionsBuilder.UseLoggerFactory(loggerFactory) .EnableSensitiveDataLogging() .UseSqlServer("Server=JOYDIP;Database=MSSQLTips;Trusted_Connection=True;"); } }
Creating a new record using the DbContext
Lastly, you can write the following code in your controller class to create a new Author record and see the console log in Visual Studio.
using (var context = new MSSQLTipsContext()) { var author = new Author() { FirstName = "Joydip", LastName = "Kanjilal" }; context.Add(author); context.SaveChanges(); }
Figure 1 shows how the console log output looks like in Visual Studio.

Figure 1: Viewing the Console Log in Visual Studio IDE
Next Steps
- Entity Framework Core integrates nicely with the logging mechanism of ASP.NET Core. For further information on this topic, you can refer to Julia Lerman’s great article here.
About the author
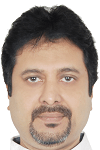
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips