By: Adam Bertram | Comments | Related: > Stored Procedures
Problem
A common task many Database Administrators (DBA) perform on a regular basis is working with SQL Server stored procedures. Stored procedures allow the DBA to automate a certain task by bundling up a query and executing as a single set of logic. This is considerable progress in including more automation, but why not automate the automation? To create a stored procedure a DBA might go into SQL Server Management Studio (SSMS), click through the GUI and create a stored procedure that way or, likewise, when executing a stored procedure pursue the same path. This is a waste of your precious time! Let's automate that.
Solution
What I mean by "automating the automation" is building a tool in PowerShell that allows us to retrieve stored procedures for review and execute them all from the command line. Among many other benefits, this gives us the advantage of then being able to add these commands to larger automation projects down the road. Let's see how we can build this tool and show how it works.
First of all, we'll need to download and install a few prerequisites if you don't already have SSMS installed. To speed things up below is some PowerShell code that downloads and installs each of the required packages to make our tool work. This code will download each MSI package in your user temp directory and run the install on each.
$files = [ordered]@{ 'SQLSysClrTypes.msi' = 'http://go.microsoft.com/fwlink/?LinkID=239644&clcid=0x409' 'SharedManagementObjects.msi' = 'http://go.microsoft.com/fwlink/?LinkID=239659&clcid=0x409' 'PowerShellTools.msi' = 'http://go.microsoft.com/fwlink/?LinkID=239656&clcid=0x409' } foreach ($file in $files.GetEnumerator()) { $downloadFile = (Join-Path -Path $env:TEMP -ChildPath $file.Key) if (-not (Test-Path -Path $downloadFile -PathType Leaf)) { Invoke-WebRequest -Uri $file.Value -OutFile $downloadFile } Start-Process -FilePath 'msiexec.exe' -Args "/i $downloadFile /qn" -Wait }
Now that you've got the prerequisites downloaded, we can begin designing our tool.
To build a great PowerShell tool, it's important to make the code inside as generic as possible while creating input parameters in a way that encourages reuse. This means bringing out items that might change over time and building in as parameter rather than a static reference. In this instance, this will be things like the SQL Server instance name, database name, stored procedure name and so on. Let's first start off with a function to run a stored procedure. This function (as well as all the following functions we'll be building) all have the appropriate parameters.
function Invoke-SqlStoredProcedure { [CmdletBinding()] param ( [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$ServerName, [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$Database, [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$Name, [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [pscredential]$Credential ) begin { $ErrorActionPreference = 'Stop' } process { try { $connectionString = New-SqlConnectionString -ServerName $ServerName -Database $Database -Credential $Credential $SqlConnection = New-SqlConnection -ConnectionString $connectionString $SqlCmd = New-Object System.Data.SqlClient.SqlCommand $SqlCmd.CommandType=[System.Data.CommandType]’StoredProcedure’ $SqlCmd.CommandText = $Name $SqlCmd.Connection = $SqlConnection $SqlAdapter = New-Object System.Data.SqlClient.SqlDataAdapter $SqlAdapter.SelectCommand = $SqlCmd $DataSet = New-Object System.Data.DataSet $SqlAdapter.Fill($DataSet) } catch { $PSCmdlet.ThrowTerminatingError($_) } } }
Most of this code is available with SMO when you install the SQLPS module. However, notice the references to New-SqlConnectionString and New-SqlConnection. Those are custom functions I've chosen to break out. Why? Because we're not only going to build this function but also one to retrieve a list of all stored procedures that exist on a server. With these standard commands broken out into other commands, they can be shared. I won't bore you with the details on them but if you're interested, feel free to take a look at the module I've created on Github.
Now notice the other function that I've created to list all of the stored procedures on a SQL server.
function Get-SqlStoredProcedure { [OutputType([Microsoft.SqlServer.Management.Smo.StoredProcedure])] [CmdletBinding()] param ( [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$ServerName, [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$Database, [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [pscredential]$Credential ) begin { $ErrorActionPreference = 'Stop' } process { try { [System.Reflection.Assembly]::LoadWithPartialName('Microsoft.SqlServer.SMO') | out-null $connectionString = New-SqlConnectionString -ServerName $ServerName -Database $Database -Credential $Credential $sqlConnection = New-SqlConnection -ConnectionString $connectionString $serverInstance = New-Object ('Microsoft.SqlServer.Management.Smo.Server') $sqlConnection $serverInstance.Databases[$Database].StoredProcedures } catch { $PSCmdlet.ThrowTerminatingError($_) } } }
Notice here I can also refer to these shared functions since making that initial connection is a common attribute both share. This is a best practice when building a tool like this. It's important to first mentally think of what you're going to accomplish before you start. In our case, it was invoking a stored procedure and listing them on a server. Then, as you begin to build your tool notice code they might have in common and break those out into other functions.
For the final test, let's take our tool for a test run. I have a SQL database in Azure to try this out on. First, I'll try to query all of the stored procedures in my myazuredatabase database on that server.
Get-SqlStoredProcedure -ServerName adbsql.database.windows.net -Database myazuredatabase –Credential (Get-Credential)
After supplying my SQL username and password you should see something like this:

This begins to enumerate each stored procedure and display each stored procedure's properties.
I know that I have a stored procedure called uspLogError in this database. Let's run Invoke-SqlStoredProcedure and see if that works as well.
Invoke-SqlStoredProcedure -ServerName adbsql.database.windows.net -Database myazuredatabase -Name dbo.uspLogError -Credential (Get-Credential)

Great! After providing my username and password again, you can see that it returns whatever the stored procedure is designed to. In this case, it was merely 0.
You can see that building a tool in PowerShell can save you a ton of time. By using this at the console to make ad-hoc queries or incorporating it into a bigger orchestration process, you'll save tons of time to get back to doing more important things.
Next Steps
- Check out the following resources:
About the author
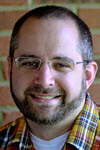
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips