By: Eduardo Pivaral | Comments | Related: > Azure Data Studio
Problem
Azure Data Studio's (ADS) popularity is growing because it is a cross-platform tool focused on working with data related tools (not just SQL Server, as support for other databases is being added over time). It provides a great T-SQL editing environment, to work with data on-premises or Azure and includes Git integration.
This tool is open source, lightweight and fully customizable. You can create custom code snippets (as I explain on this tip), change the color theme on the fly, create data insights, create SQL Notebooks (as I explain on this tip) and a lot of other options to make the tool suit your very unique needs.
Among those customizations, you can create your own extensions (review this tip to learn more about ADS extensions), so you can share with your team or the community your own tools for easy deployment.
If you are new to developing code, it can be difficult to know where to start, but with the tools available, you can create your own extension very easily.
Solution
There are different types of ADS extensions, but in this tip we will focus on creating a TypeScript extension, with this type of extension you can create wizards, message boxes, contextual menus or any functionality you want to add to it.
If you don't have Azure Data Studio yet, you can download the latest version here.
Please note that this tip is not intended to show you how to program in TypeScript language, but is an introduction on how you can implement your code into ADS functionality.
You can check the TypeScript official site here, once you are comfortable with this language, you can start creating your own extensions with this guide.
In this tip we will show you how to display server information for the active connection in ADS, how to test it and how to create a .VSIX file to share your extension.
Prerequisites to create an Azure Data Studio Extension
In addition to having Azure Data Studio up and running on your machine, you need some other tools to create an extension.
You need to download and install the following:
- Node.js
- A JavaScript runtime, you can download the latest version here. Make sure that during install, you select the add to PATH option, also include npm (Node.js Package Manager) in the installation options, it will be used to install the extension generator and package creator.
- Visual Studio Code (VSCode)
- It will be used to code and debug your extension, you can download it from here.
- Azure Data Studio Debug Extension
- From your VSCode IDE, search and install that extension, also you can download it from here. It will allow VSCode to have a runtime debug for the extension while running an ADS instance.
Once you have download and installed all of the above, we are ready to setup our environment.
Preparing our environment
Open VSCode and open a new terminal (from the command palette or the menu):

Once the terminal is open, select CMD terminal shell, we will install the Yeoman extension generator using npm with the following command:
npm install -g yo generator-azuredatastudio
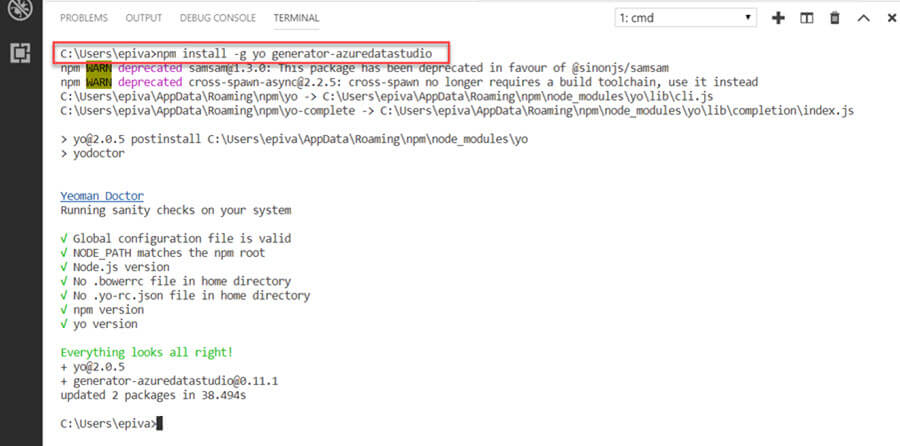
For packaging your extension into a .vsix file, you need to install vsce using npm. In the same terminal, run the following code to install the vsce package:
npm install -g vsce

And with that, our environment is ready, we can start creating extensions.
Creating the Azure Data Studio Extension
Using the terminal from VSCode, we will use the Yeoman Azure Data Studio extension generator to create an extension template.
To run the generator, use the following command:
yo azuredatastudio
A list of options will display, you can see there are different templates, for this example we will create a TypeScript extension, using the arrows select New Extension (TypeScript):
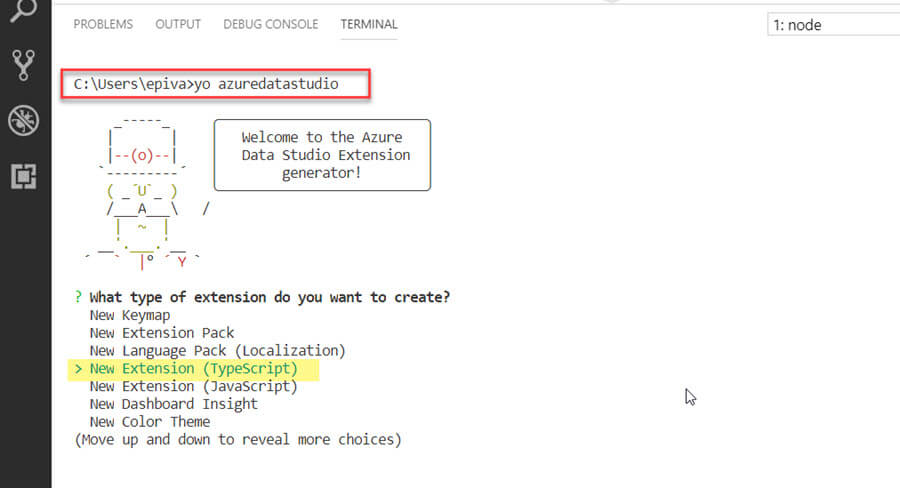
After selecting the extension type, some questions will appear, fill those with the information you want. It should look like this once complete:
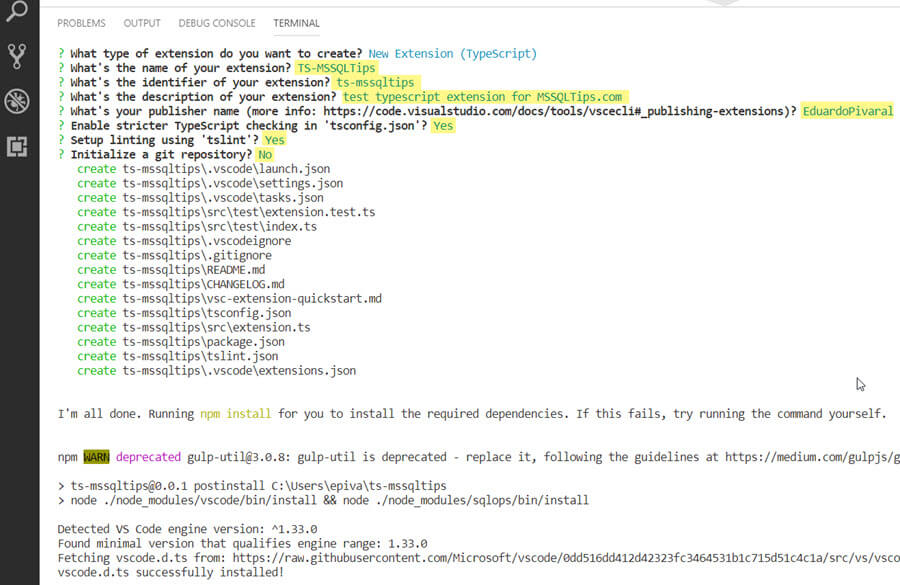
Wait some seconds and the template will be created, now we can start working on it. For this, navigate to the folder created by the generator and access it, using the following command:
cd [yourextensionfolder]
And then access it using the following command:
code .
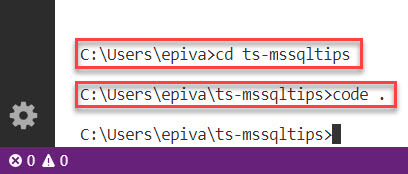
A new VSCode instance will open with the template generated:
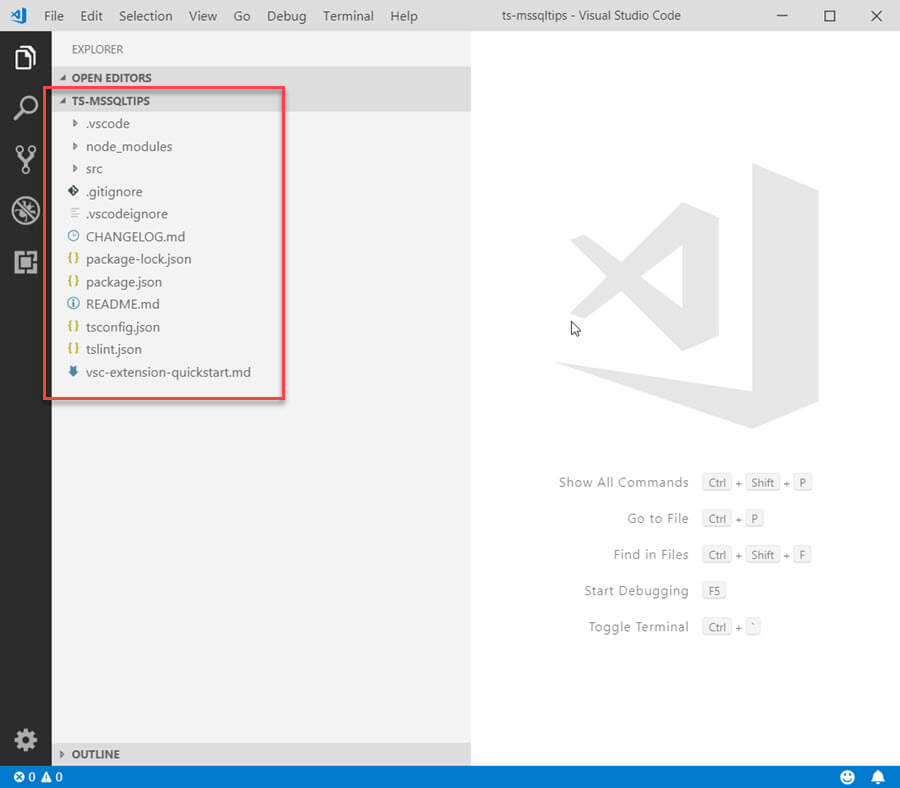
We will start checking the events we can configure and their respective actions. For this, open package.json file, you will see that the template has generated a hello world and will show an active connection by default:
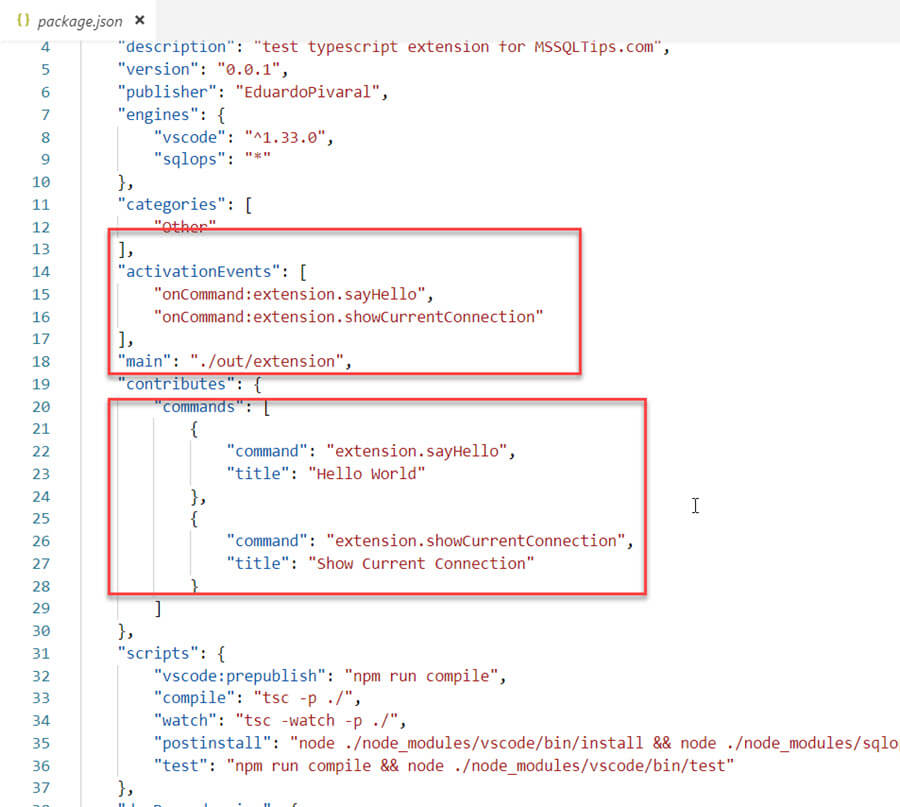
The activation events tell us what will trigger the command execution, and what command must be executed. If you want to check all available activation events, check VSCode documentation here.
The default behavior OnCommand indicates that the command must be invoked from the command palette (we will see this later when we debug the extension).
The Commands section tells us the command to execute for each action and the display name for each command.
If you want to see the template in action, you could debug the extension right now and you should be able to see the hello world command in action, but for this tip we will change the default command now and debug it later.
Our first step is to change the commands to execute, we will leave just one command and one activation event, so we replace the code shown above with this:
"activationEvents": [ "onCommand:extension.ShowServerInfo" ], "main": "./out/extension", "contributes": { "commands": [ { "command": "extension.ShowServerInfo", "title": "Show Server Info" } ] }
It should look like this:

Now, we will define the code for the command we have defined. For this, open the extension.ts file, you will see the code generated from the template, that shows us the hello world message box:
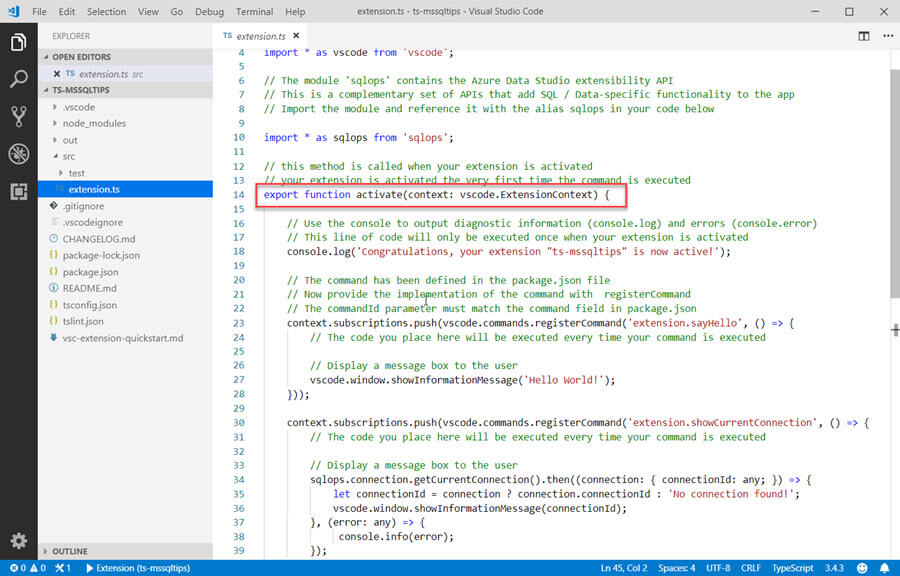
You will see that the code generated has some comments as well that can guide you on what each section of code does. We will focus on the activate function, so delete all the contents inside this function and replace it with this code:
export function activate(context: vscode.ExtensionContext) { //registering the command we defined on package.json let disposable = vscode.commands.registerCommand('extension.ShowServerInfo', async () => { // use async call to get the connection info let connection = await sqlops.connection.getCurrentConnection(); if (!connection) { let allConnections = await sqlops.connection.getActiveConnections(); if (allConnections && allConnections.length > 0) { connection = allConnections[0]; } } // Format the message let message = 'No active connection!'; if (connection) { let connInfo = await sqlops.connection.getServerInfo(connection.connectionId); message = 'Server name:' +connection.options['server']; message += ' SQL Version: ' + connInfo.serverVersion; message += ' Is Cloud?: ' + connInfo.isCloud; } // Display a message box to the user vscode.window.showInformationMessage(message); }); context.subscriptions.push(disposable); }
With this code we will obtain the current connection, and display some basic information about the server (name, version and if is cloud or not), via a message box to the user.
The contents of the file should look like this (you can modify the code if you want):

At this point, we are ready to debug our extension to see our progress so far.
Debugging our Azure Data Studio Extension
Depending on the generator and debugger version you are using, you can encounter an issue with the debugger. To make sure the configuration is correct, select the .vscode folder and then open launch.json file.
Check the "runtimeExecutable" property, if it has "sqlops" value on it, it will generate an error when starting the debugger.
To fix this, just change the property value to "azuredatastudio".
It must look like this:
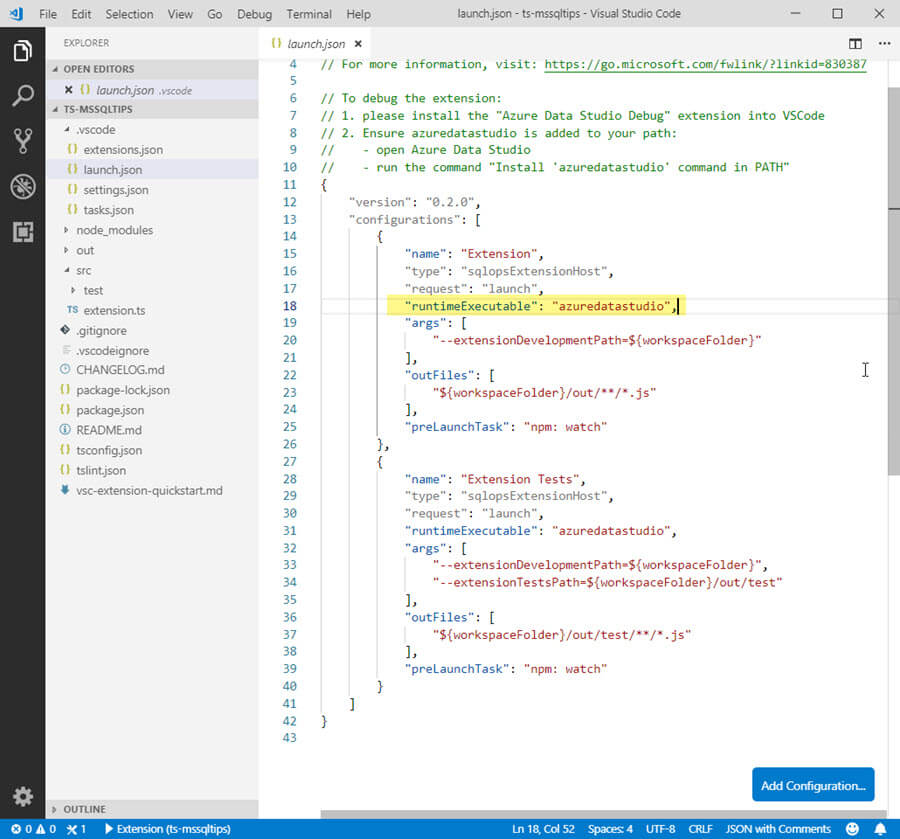
Once you have checked this value is correct, you can start the debugger. Select the option from the menu or press F5:

A new instance of Azure Data Studio will open, for this example I will connect to a local instance and to an Azure instance:
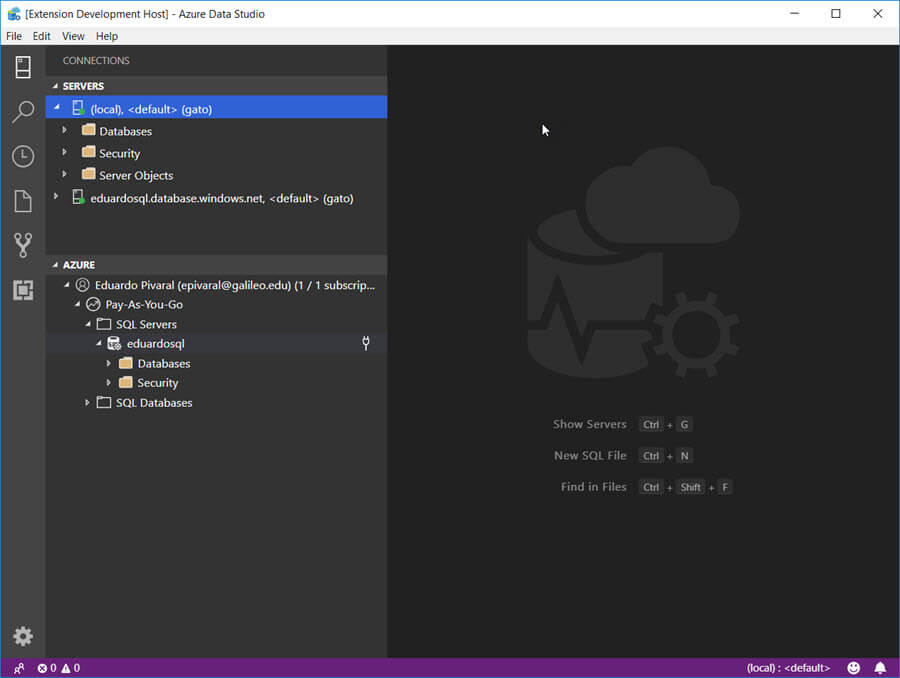
First, we select the local instance, and then select the Command Palette (F1 or Ctrl + Shift + P), then type the title you gave to your command, in our case Show Server Info:
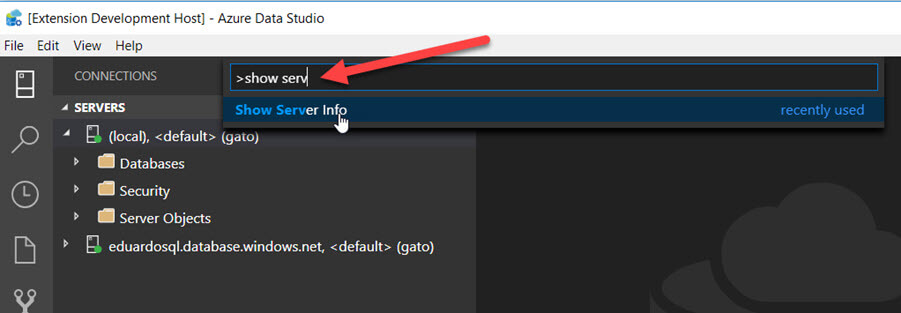
A Message box is displayed on the bottom right corner of our ADS window with the information we coded to show:
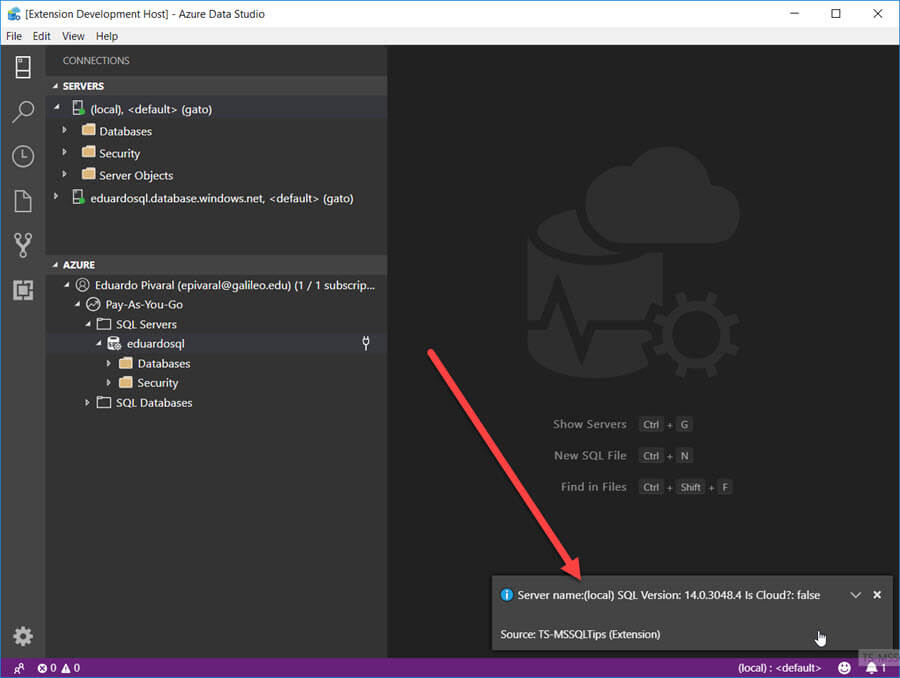
Let's check the command again, this time selecting our Azure Database:
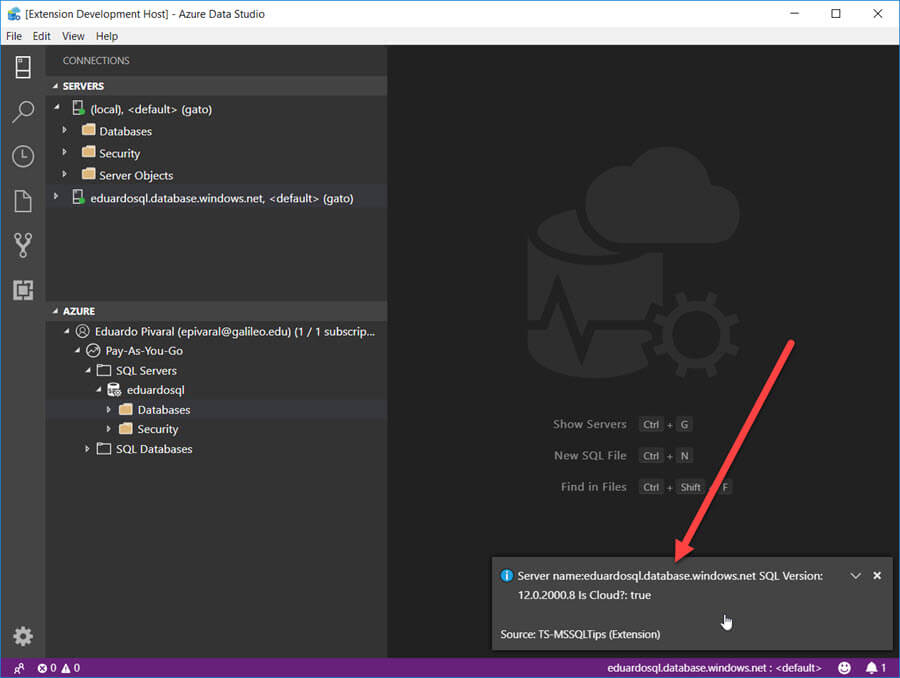
The proper information has been displayed.
We have tested the code successfully, now we will add additional functionality to our code, being able to call our command from a contextual menu (right clicking on the server).
Calling a Contextual Menu in Azure Data Studio
For our next example, we will allow the function we previously created to be called from a contextual menu.
To do this, we need to modify the package.json file. Open the file and just below the commands property, separated by a comma, we add the following piece of code:
"menus": { "objectExplorer/item/context": [ { "command": "extension.ShowServerInfo", "when": "connectionProvider == MSSQL && nodeType == Server", "group": "NONE" }] }
Explaining the code: we will bind our command extension.ShowServerInfo to the object explorer context menu, when the connection selected is SQL Server.
The group property is used if you want to group multiple options on the same section. In our case we select NONE since we want our command in its own section.
You can learn more about the context menu properties checking official documentation here.
The final code should look like this:
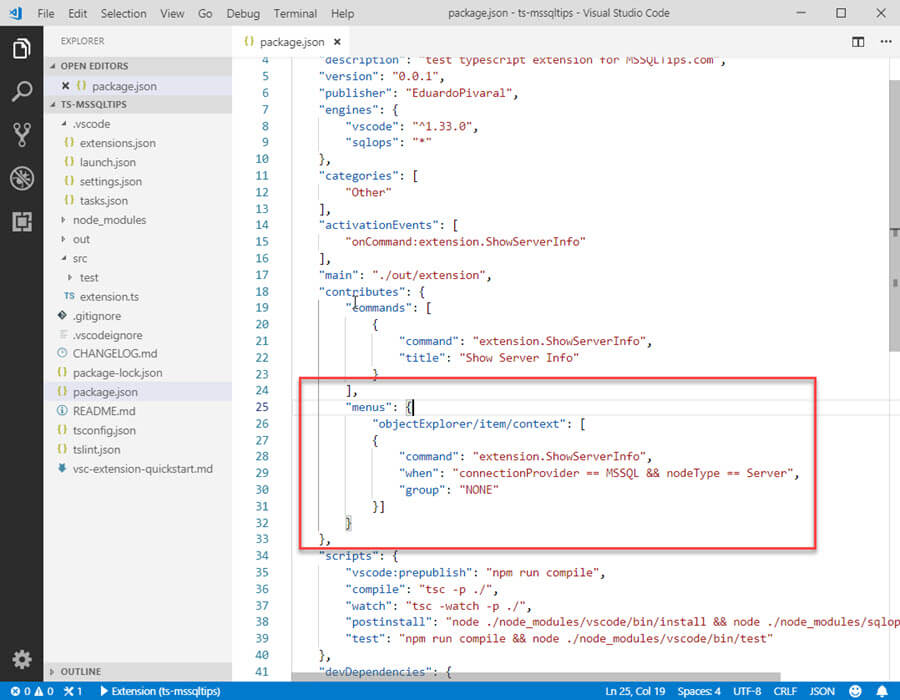
If we debug our extension again, you will see that now we are able to call our function by right-clicking on a server:
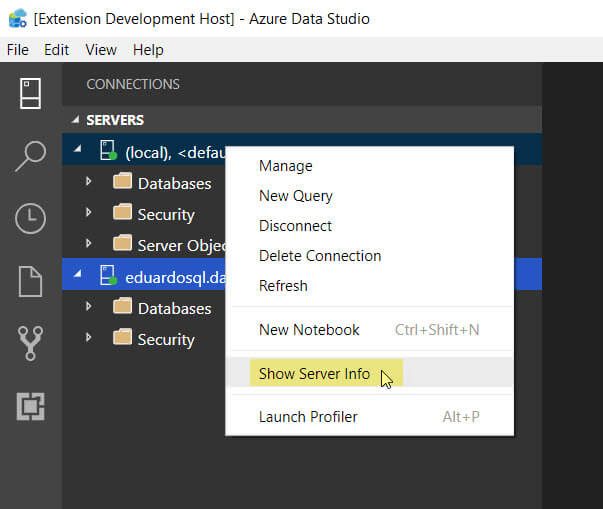
Once you are happy with the results, we can package our extension in a .vsix file to be distributed.
Creating a .VSIX file in Azure Data Studio
To create a .vsix file, some additional modifications must be made, so the vsce utility does not give us any errors.
First, modify the README.md file (using the Markdown language), a validation is made to make sure this file has been updated, just add some useful information:

Then open the package.json file and add a "repository" property, for this value you can put a valid GitHub URL or leave the value blank.
In the engines property, modify the vscode value to a lower one (for example ^1.20.0) so you don't have any issues when importing .vsix file to Azure Data Studio, since the VSCode build is checked at import time and can trigger an error if this value is higher than the vscode build of the ADS version you are importing the extension.
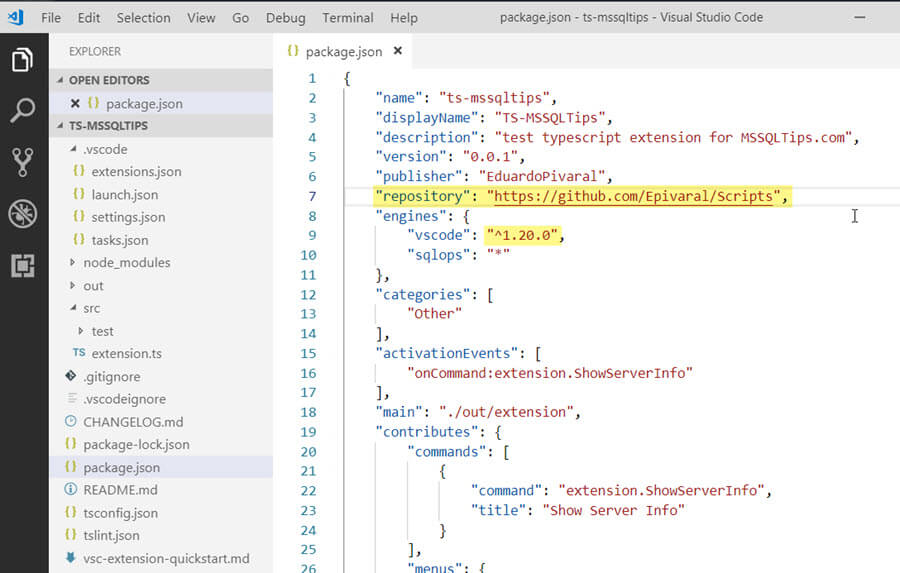
Now we are ready to create our extension package.
Open a terminal and run the following command:
vsce package
If everything is ok, our .vsix extension will be created in the same folder:

And that is all, then you can distribute and have other users import the .vsix file using the import option in Azure Data Studio:

Now the extension has been installed successfully:

Next Steps
- This example is on a Windows PC, but since all the tools used are cross-platform, so you can work through the same steps on a Linux or MacOS environment.
- Check my previous tip on how to create custom snippets on Azure Data Studio.
- Check my previous tip on how to create SQL Notebooks on Azure Data Studio.
- An excellent Markdown language reference can be found here.
- You can find out more about VSCode extension here.
- You can download the latest Azure Data Studio release here.
About the author
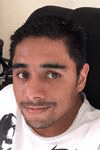
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips