By: Hristo Hristov | Updated: 2022-03-07 | Comments | Related: > Python
Problem
Python is a multi-purpose programming and scripting language well suited for the data professional. The readability and expressivity of the Python syntax are second to none and allow for a less steep learning curve. Frequently in your script you will want to steer the program execution to different blocks of code. This process is called directing the control flow.
Solution
To master control flow in Python you need to be familiar with the comparison rules and the if-else commands. Knowledge of these two concepts will allow you to direct the control flow of your program as needed. Python's conditionals do have some caveats as compared to other programming languages. We will show these caveats here, so let us dive in.
Comparisons with Python Programming
Before we start with if-else statements, let us recap how comparing values works in programming from the perspective of the core values and the operations between them.
You compare values with:
- operator < (less) or <= (less than or equal to)
- operator > (more) or >= (more than or equal to)
- operator == (equal to)
- operator != (different from)
print(1 == 2) print('a' == 'a')
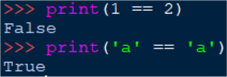
Additionally, we also have the following operators that are handy when doing checks between (custom) data types or membership checks:
The operator is
:
is
tests object identity, i.e., is one variable identical to another
one. For example, here are two identical variables. Both tests (is
for identity and ==
for equivalence) will output True
:
a = 'MSSQL_tips' b = 'MSSQL_tips' a is b a == b

In fact, these two variables are one variable, as the memory pointer points to the same address in memory. Here is how to check if that is the case:
hex(id(a)) hex(id(b))

However, examine this case:
my_list = [1,2,3] another_list = [1,2,3] my_list is another_list my_list == another_list
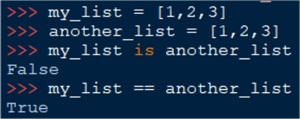
The reason we got False
on the identity comparison
here is because that the two lists are mutable, so Python has assigned two different
addresses in memory to store the same values:
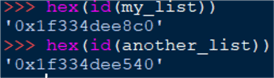
The operator in
:
in
tests for membership, i.e., is a value present in (or part of)
another value. Usually we test against a collection, although strings can be tested
for membership too:
my_str = 'MSSQL' 'SQL' in my_str # another check my_list = [1,2,3] 1 in my_list

As seen in the examples so far, the outcome of a comparison is a Boolean result which can take only two values – true or false depending on whether the outcome of the comparison is right or wrong.
Logical "and" and "or"
In Python there are:
- three Boolean operators:
and
,or
andnot
- two Boolean values:
True
andFalse
Here is what expressions involving these operators and values will return when you use them:
True and False True or True True or False not True not False

With these foundations covered, let us move on to explaining how conditional statements in Python work.
If Statement
The if block is a fundamental Python construct that allows you to control the flow of your program.
How to Initialize
Initializing is straightforward. You start with the if
keyword:
if conditional: # do smth elif conditional: # do smth else else: # do a default thing
The elif
statemetns which follow, will normally refer to additional conditions
you want to get tested as part of your overall conditional statement block. There
is no limit how many elif
's you can use (within reasonable limits of
course). Finally, you write the else
block, which is a fallback for
all cases that were not covered by the opening if
and preceding
elif
's.
Note how the code blocks under the if
, elif
and
else
clause are all situated at the top-level in terms of indentation. Indentation
in Python helps you differentiate between different blocks of nested code:
# code block 0 If a == b: # code block 1 If c == d: # code block 2

The good practice is for the indentation to consist of a tab or four spaces. It must be consistent across the script otherwise you may get unexpected or inconsistent indentation errors.
Nested conditional checks
Of course, conditional statements can be nested under each other. Check out the following example:
if number >= 0: if number < 100: print('two digit number') elif number >= 100: if number < 1000: print ('three digit number') else: print('unknown') else: print('negative number')
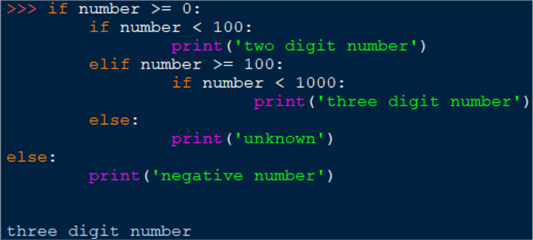
However, a generally better, more efficient, and readable way to do the same would be the following code:
if number >= 0 and number < 100: print('two-digit number') elif number >= 100 and number < 1000: print('three-digit number') else: print('negative number')

Combining the conditional checks is called chaining. Chaining allows you to group
your checks on the same line improving readability. Bottom line, try to avoid nesting
conditional statements if possible. Use chaining by combining the predicates into
one statement and passing it after the if
and elif
conditions.
Thus, your code becomes easier to follow and comprehend.
Using parenthesis
Occasionally, conditions become more complex and will need to be additionally logically grouped. You can perform this grouping with the parenthesis operator. Not only does it help visually, but just like in math it defines the operation execution priority. Let us take an example with a rectangle, drawn in 2D space:

This rectangle is defined by point A with coordinates x1 = 0, y1 = 0 and point B x2 = 4, y2 = 2. Let us imagine we wanted to check if a random point E defined by coordinates x, y is inside the rectangle. We need to construct a statement like this:
x1 = 0 y1 = 0 x2 = 4 y2 = 2 x = 1 y = 1 if x > x1 and x < x2 and y > y1 and y > y2: print('inside')

We have chained four statements to form our condition. It is, however, beneficial to group the already chained statements by using parenthesis:
if (x > x1 and x < x2) and (y > y1 and y > y2): print('inside')

Grouping with parenthesis helped, but we can streamline this statement further. What I would strongly recommend is to define variables for your conditional statements. This way you make each condition a separate unit of code that is easier to track and debug. For example:
inside_x = x > x1 and x < x2 inside_y = y > y1 and y < y2 if inside_x and inside_y: print('inside') else: print('outside')

With the given values for the points, the result is "inside".
Ternary expression
Additional to the canonical if-else statement, there is the ternary expression.
This construct allows you to create an expression that will return the same result
as an if
statement. If you had a piece of code like this:
tip = 'some text' if 'SQL' not in tip: print('boring') else: print('great')
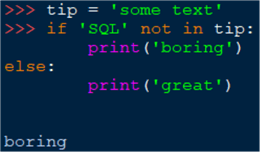
You can get the exact same result by converting the if-condition statement into a ternary expression:
result = print('boring') if 'SQL' not in tip else print('great)

Notice also how we use the not in
keywords to define our condition
in both the if-statement and the ternary expression. For some engineers, the intuitive
approach would be to state if 'SQL' in tip:
and then proceed accordingly.
If you want to exercise a bit more, then try the other way around by negating your
condition like we did here!
Switch-case
Python does not have a switch-case operator per say, as C# does for example. This operator can be handy when you have lots of options (e.g., more than five) and you would like to optimize the code a bit instead of using a big sequence of elif-s. Here though we have match-case to help. Defining it looks like this:
match subject: case <pattern_1>: <action_1> case <pattern_2>: <action_2> case <pattern_3>: <action_3> case _: <action_wildcard>
Here is an example:
def http_error(status): match status: case 400: return "Bad request" case 404: return "Not found" case 418: return "I'm a teapot" case _: return "Something's wrong with the internet"

There are also ways to "emulate" the match-case operator. Let us look at one of them by using a dictionary and a lambda function:
options = {1:'option1',2:'option3',3:'option3'} >>> f = lambda x: options[x] >>> my_var = 2 >>> f(my_var) 'option3'

By using a mapping data type, such as the dictionary, we are adding the outcomes of our checks as the values to the keys in the dictionary. The keys themselves are just indices pointing to the right outcome from the conditional check. With a lambda function, it is then easy to perform our check. For a refresher on lambda functions, please check the previous tip in the series.
Conclusion
This tutorial provided an overview of how to develop and use Python conditional statements
(if-elif-else) and the ternary expression in the above code. We also discussed the basic of comparisons
in programming with the operators and
, or
and not
as well as the Boolean values True
and False
.
Next Steps
More Python Articles and Python Tutorials
- Why use Python?
- How to Get Started Using Python using Anaconda, VS Code, Power BI and SQL Server
- Python Basic Built-in Data Types
- Learn Python Complex Built-in Data Types including List, Tuple, Range, Dictionary and Set
- Python List Comprehension for Lists, Tuples, Dictionaries and Sets
About the author

This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips
Article Last Updated: 2022-03-07