By: Hristo Hristov | Updated: 2022-10-13 | Comments | Related: > Python
Problem
The Python programming language has important "reserved" commands called keywords. Each one of them provides specific special instructions for the interpreter. Using the keywords comes with certain caveats, and it is handy to have a complete overview of their use.
Solution
This Python tutorial provides a listing and overview of all Python keywords. These keywords are categorized according to their function: Boolean, conditionals, logical operators, membership checks, exceptions, and loops, along with explanations and examples. Note: These are only the keywords; built-in methods or other methods from external libraries are not discussed.
Python Keyword Overview
Here is the list of all keywords in alphabetical order:
- and
- as
- assert
- async
- await
- break
- class
- continue
- def
- del
- elif
- else
- except
- False
- finally
- for
- from
- global
- if
- import
- in
- is
- lambda
- None
- nonlocal
- no
- or
- pass
- raise
- return
- True
- try
- while
- with
- yield
Let's group them functionally, explaining what each of them does and how they work.
Boolean
True False
These are the ubiquitous Boolean values. Unlike other programming languages,
both are capitalized. The evaluation result of directly comparing two variables
(or testing them for equivalency) will return either True
or False
.
a = 'SQL' b = 'MySQL' print(a != b) print(a == b)
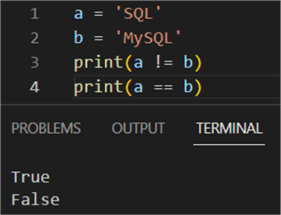
Additionally, a variable can be assigned to the True
or False
value:
a, b = True, False print(a, b)
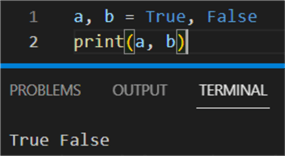
Finally, you can assign the actual check for a value to a variable. This variable
will return True
or False
.
In this case, we check if the value of a
is a certain
integer. With the help of the and
,
or
, and not
keywords, this technique can help to construct complex logic checks:
a = 1 c = a == 2 print(c)

Conditionals
These keywords are vital in constructing control flow logical checks.
if elif else
The elif
is short for else if. The two most common
ways to use these keywords are in if-else
or
if-elif-else
statements, where the
elif
may be repeated as many times as branches are
needed.
Here is a simple example:
a = 0 if a == 1: print('Variable a has value of 1') elif a == 2: print('Variable a has value of 2') else: pass
Note: The placeholder value pass
under the
else
clause: The program will not do anything here,
but will not pause.
Another way to use the if-else
conditional statement
is by using only the if
clause. This sometimes occurs
when you check for a specific value of a variable without any other branches. A
common use of this technique is in a for-loop:
for i in range(10): print(i) if i == 3: print('Level 3 reached')

Finally, check the ternary use of if-else
in my
tip,
Python Control Flow Logic including IF, ELIF and ELSE. While it is convenient
as a one-liner, I would not recommend it for nested checks.
Logical Operators
These are logical operators.
and or not
The and
operator accepts multiple expressions which
evaluate to True
or False
and returns one Boolean value as a result. If any of the inputs is
False
, the result will be False
.
With or
, it is enough for one of the inputs to be
True
for the whole result to be
True
. On the other hand, not
is the logical negation operator. It is commonly used to reverse the value of a
Boolean variable, i.e., a True
variable will become
False
if negated with not.
Here is a tabular overview:
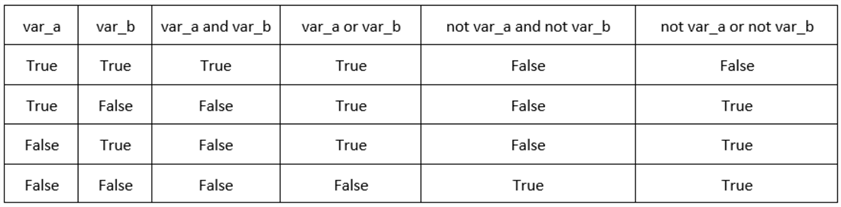
Membership Check
This keyword has two functions. The first is to perform a membership check against an iterable.
in
Here is an example that also makes use of not
in combination with in
:
my_var_a = 'a' my_var_d = 'd' sequence = ['a', 'b', 'c'] if my_var_a in sequence: print(f'Variable {my_var_a} present.') if my_var_d not in sequence: sequence.append(my_var_d) print(f'Variable {my_var_d} added.')
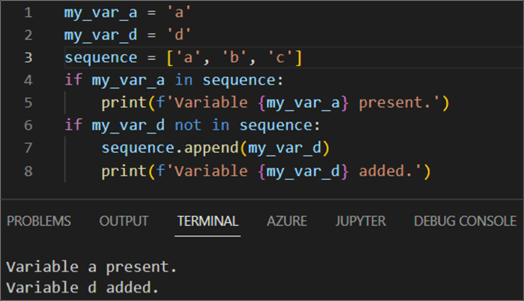
The second function of in
is iterating over a sequence
in a for loop, for example:
for i in range(3): print(i) # prints 0, 1, 2
range
generates an immutable sequence; therefore,
in
can be used with it.
Exceptions
These are the keywords related to developing robust code that expects errors might arise and is prepared to handle them gracefully.
try except finally raise
The main clause,
try
, "tries" the code block for an exception.
except
will raise one or more exceptions depending
on the error that might have occurred. The code under finally
will execute regardless of if an exception was raised or not. The
else
clause can also be used prior to
finally
to execute a code block only if no exception
occurred. I have written an extensive tip on exception handling called
Python Try Except Code Samples.
The with
keyword is a special case but still belongs to the
exceptions section.
with
It is a generalization of a lengthier code that would look like this:
try: # do smth finally: # clean-up
The general syntax of with
is:
with expression [as variable]: with-block
The expression must support the context management protocol, which implements
special __enter__
and __exit__
methods. The former ensures that whatever we do is set up correctly, and the latter
ensures that even if an exception is raised, clean-up will be performed.
In practice,
there are two main situations when you will use with
:
1 - File Objects
with
enables us to quickly set up read or write blocks of code with little effort. In
this example, file.txt contains three lines of text:
path_to_file = r'C:\Users\file.txt' with open(path_to_file, 'r') as f: read_data = f.read() print(read_data)
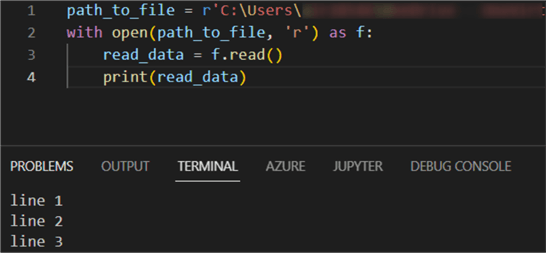
With the help of
with
, here we have ensured that the target file is
handled efficiently while there were data lines to be retrieved.
2 - Context Managers
A database connection is a context manager; therefore, it can be used in a with-statement:
import pyodbc connection_str = 'DRIVER= {ODBC Driver 17 for SQL Server}; \ SERVER=.; \ DATABASE=AdventureWorks2019; \ Trusted_Connection=yes' query = 'SELECT TOP 100 * FROM Person.Person' with pyodbc.connect(connection_str) as connx: cursor = connx.cursor() cursor.execute(query) data = cursor.fetchone() print(data)
The output is the first result of the query (because of
the fetchone
method):

An extensive tip, How to Query SQL Data with Python pyodbc, explains how to use the pyodbc library.
For Loops and While Loops
In this next category, we arrive at all loop-related keywords:
for while break continue
for
and while
are
used to construct a loop. The loop is a construct that allows repeating a block
of code according to a predefined rule or condition. For example, with
for
and enumerate
, we
can easily go over every element from a sequence together with its index:
my_list = ['a','b','c'] for i, v in enumerate(my_list): print(i,v)
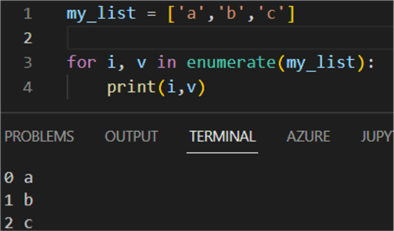
On the other hand, while
enables us to construct
a loop that executes only when a certain condition is true. For example, if we take
a target number, we can decrease it and display if every single number less than
or equal to it is odd or even:
my_num = 10 while my_num > 0: if my_num % 2 == 0: print(f'The number {my_num} is even') else: print(f'The number {my_num} is odd') my_num -= 1

With while
, you must always beware of the risk
of designing a runaway loop. In the example above, forgetting to decrease
my_num
by one will create such a loop.
Next is the break
keyword. Its core usage is when
you want to terminate a loop's execution if a certain condition has been reached.
For example, we can "break" the previous loop if our number gets to
7:
while my_num > 0: if my_num == 7: break if my_num % 2 == 0: print(f'The number {my_num} is even') else: print(f'The number {my_num} is odd') my_num -= 1
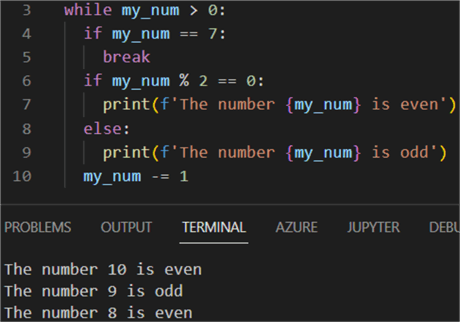
Therefore, the loop exits as soon as the variable my_num
becomes 7.
Unlike break
, continue
will allow the loop to proceed but skip a certain action. Using the same example,
with continue
, we can skip the number 7:
while my_num > 0: if my_num == 7: my_num -= 1 Continue if my_num % 2 == 0: print(f'The number {my_num} is even') else: print(f'The number {my_num} is odd') my_num -= 1
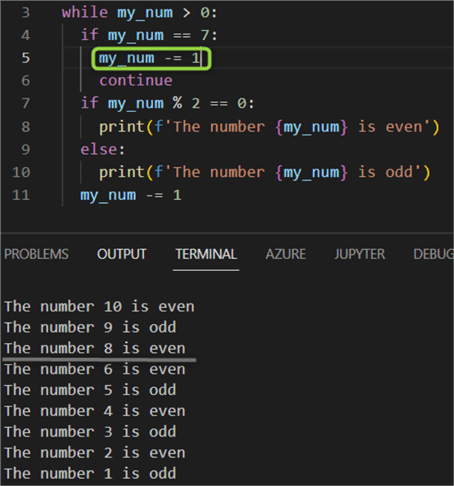
However, we must still decrease the my_num
variable
by one; otherwise, we will create an infinite loop.
Conclusion
This tutorial examined Python keywords related to Boolean variables, conditional expressions, logical operators, membership checks, exception handling, and loop construction. This was the first one of a two-part subseries on Python keywords. The next tip will examine the keywords related to modules, classes, functions, coroutines, and others.
Next Steps
- Python keywords
- Python with explained
- Learn Python Control Flow Logic including IF, ELIF and ELSE
- Learn Python Loops Including FOR, WHILE, Nested and more
- Python Try Except Code Samples
- Learn Python Loops Including FOR, WHILE, Nested and more
About the author

This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips
Article Last Updated: 2022-10-13