By: Nai Biao Zhou | Updated: 2023-03-29 | Comments (1) | Related: > Python
Problem
We may need to share our Python desktop or console applications with business users or deploy these applications to multiple machines. However, there are some challenges in the delivery of Python applications:
- Running Python scripts requires a Python environment and all required Python modules.
- Different applications may need different versions of Python and modules.
- Some users may have the challenge of running the programs through the command line.
- Python developers do not want others to play with the source code.
To overcome these challenges, we would like to have these Python applications run without Python installed on the system. Therefore, we want to know how to make a Python application into an executable that bundles all the dependencies.
Solution
We can create a distributable executable in Python using PyInstaller. PyInstaller bundles a Python application and all its dependencies into a single package (Cortesi, 2023). Therefore, users can run Python applications without going through the steps of installing a Python interpreter or any required modules. We also look at the auto-py-to-exe module, which uses a simple graphical interface, PyInstaller, and a web browser (ex., Chrome) to perform the conversion. After creating a single folder that contains an executable file and all the requirements to run the executable, we can create a deployment package from this folder.
The article walks through the steps to convert python files to executable files. We first set up the testing environment. Next, we install the PyInstaller module. Then, the article introduces PyInstaller command line arguments. For example, we use the add-data option to add the icon file to the executable. Then, we demonstrate how to read error messages and fix an error when running the executable. Finally, we briefly explore the auto-py-to-exe module.
The author tests the solution using Python 3.10 (64-bit) on Windows 10 Pro 10.0 <X64>. The development environment has Chrome with Version 110.0.5481.178. In addition, we test the executable on a Windows 10 Home operation system with no Python installed.
1 – Setting Up the Testing Environment
We use the Python GUI application created in the tip "Creating a Python Graphical User Interface with Tkinter." We can click here to download the complete source code. Here are the files in the downloaded packages:
- MSSQLtips.ico
- MSSQLTips_GUI_app.py
- MSSQLTips_GUI_backend.py
- requirements.txt
We copy the files to the "C:\temp\MSSQLTips\" folder. We can create this folder if the folder does not already exist. We then open Command Prompt and use the CD command to set the folder as the current working folder. Next, we run the following command to create a virtual environment:
python -m venv C:\temp\MSSQLTips\myenv
Then, we enter the following command in the Windows command prompt to activate the environment:
C:\temp\MSSQLTips\myenv\Scripts\activate
Next, we use the following command to install all external dependencies that we previously recorded in the requirements.txt file:
python -m pip install -r requirements.txt
Figure 1 illustrates the execution of these three commands. At this step, we complete installing the application on a computer with a Python environment. After that, we should be able to run the GUI application successfully.
python MSSQLTips_GUI_app.py
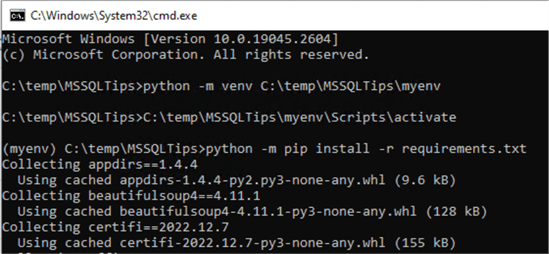
Figure 1 Create a Virtual Environment and Install all External Dependencies
2 – Installing PyInstaller
The command to install the PyInstaller module is like installing other Python modules. First, we need to make sure the virtual environment is active. Then, we enter the following command in the Windows command prompt to install the module:
pip install PyInstaller
We can run the following command to check the version of the module and ensure we install the model successfully.
pyinstaller --version
Figure 2 shows the output of these two commands. Then, we are ready to create a single package with an executable inside using PyInstaller.

Figure 2 Install the PyInstaller Module
3 – Pyinstaller Command Line Arguments
After installing the Pyinstaller module, we can convert the Python script to an executable. For example, the following command line shows the syntax:
pyinstaller MSSQLTips_GUI_app.py
When running the command successfully, we receive many lines of messages in the console window, which should look like in Figure 3. During the execution, PyInstaller reads and analyzes the Python script file, discovers all dependencies, and then bundles the script and all its dependencies into one package (Khandelwal, 2020).
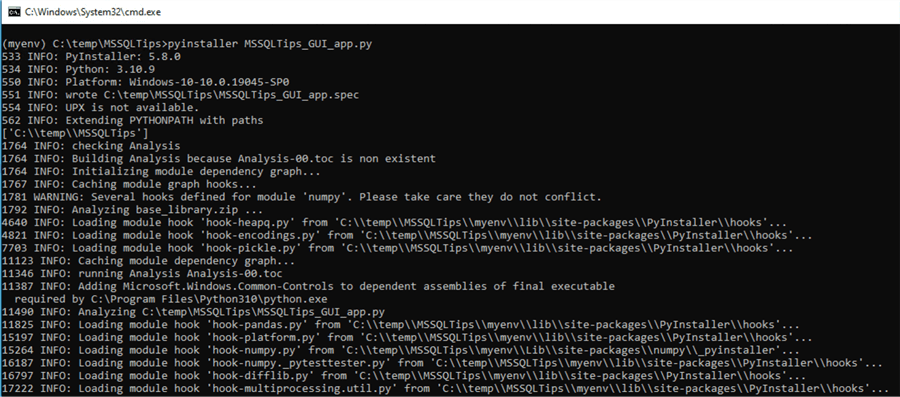
Figure 3 The Output of the Pyinstaller Command
After completing the conversion, PyInstaller creates a new file and two new folders in the current working folder, which should look like Figure 4. Using PyInstaller to generate an executable makes Python source code reading or modification harder; however, we cannot use Pyinstaller to secure the source code. Compiling the embedded code from such an executable file is difficult, but it is doable (Jaworski & Ziade, 2016).

Figure 4 The Folders and Files Generated in the Conversion Process
The new file has the same name as the python script file but with the extension ".spec," which represents a specifications file. The spec file contains information about the source files. The advanced user may modify the spec file to customize the conversion process (Khandelwal, 2020).
Each of the "build" and "dist" folders contains a subfolder, "MSSQLTips_GUI_app," which has the same name as the python script file. The "build" folder contains some log files and working files. The "dist" folder includes the main executable file with the same name as the script file. The "build" folder also contains all requirements to run the executable file (Thapliyal, 2020). We can use the "dist\ MSSQLTips_GUI_app" folder to create a deployment package.
A simple deployment method is to copy the folder to the target machines and create a shortcut of the "MSSQLTips_GUI_app.exe" file. However, we should get an error, shown in Figure 5, when running the executable. The error message indicates that the executable missed a file or directory called "VERSION." We find the "VERSION" file in the installed packages folder, as shown in Figure 6. We can manually copy the "VERSION" file to the "dist" folder; however, we want Pyinstaller to handle this automatically.
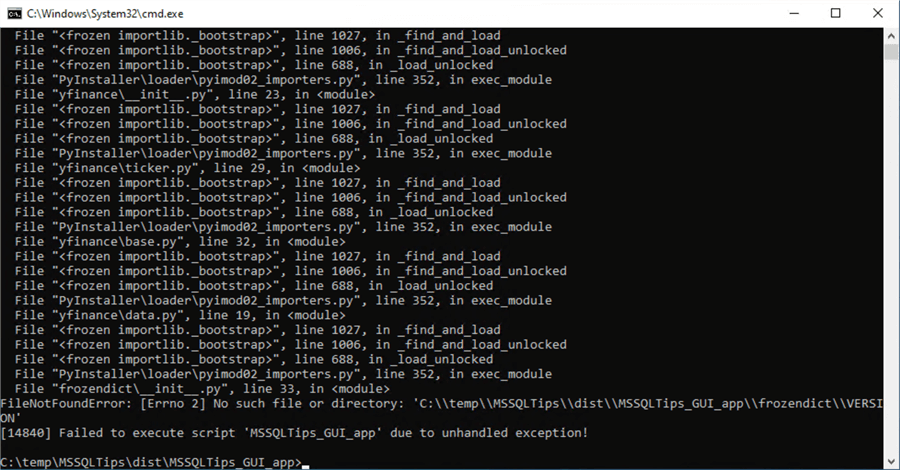
Figure 5 Failed to Run the Executable
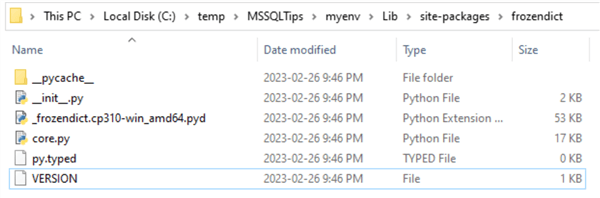
Figure 6 The Folder Structure of the frozendict Module
Pyinstaller command line arguments can add the missing file to the executable. In practice, we usually need to add data files to the deployment package (Khandelwal, 2020). Pyinstaller provides many arguments to help build the deployment package. We can use the following command to list all arguments. The output of the command should look like Figure 7.
pyinstaller --help
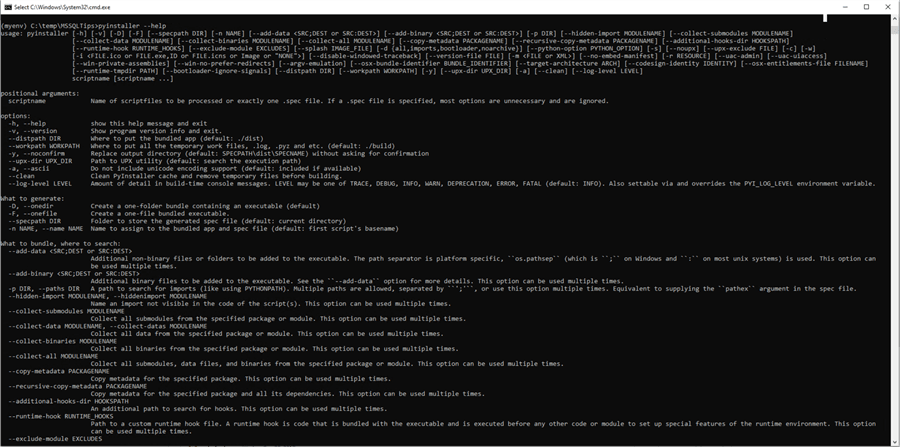
Figure 7 The Pyinstaller Help Document
Table 1 lists some common arguments on the Windows system. By default, Pyinstaller creates a one-folder bundle containing an executable. We have already seen the "dist" folder. We can create a one-file bundled executable using the "--onefile" option. However, when the Python application is as complicated as the Python GUI application used in this exercise, we recommend the "--onedir" option, allowing us to inspect all the dependencies included (Lee, 2019).
Argument | Description |
---|---|
-h, --help | Show this help message and exit. |
-v, --version | Show program version info and exit. |
-y, --noconfirm | Replace output directory (default: SPECPATH\dist\SPECNAME) without asking for confirmation |
-D, --onedir | Create a one-folder bundle containing an executable (default) |
-F, --onefile | Create a one-file bundled executable. |
--add-data <SRC;DEST> | Additional non-binary files or folders to be added to the executable. This option can be used multiple times. |
--add-binary <SRC;DEST> | Additional binary files to be added to the executable. This option can be used multiple times. |
--hidden-import MODULENAME, --hiddenimport MODULENAME | Name an import not visible in the code of the script(s). This option can be used multiple times. |
-c, --console, --nowindowed | Open a console window for standard i/o (default). On Windows this option has no effect if the first script is a '.pyw' file. |
-w, --windowed, --noconsole | Do not provide a console window for standard i/o. On Windows this option is automatically set if the first script is a '.pyw' file. |
-i <FILE.ico>, --icon <FILE.ico> | Apply the icon to a Windows executable. |
Table 1 Some Common Pyinstaller Command Line Arguments on the Windows System
4 – Creating an Executable Using PyInstaller
We want to apply the icon "MSSQLtips.ico" to the executable. We also want to add the "VERSION" and icon files to the executable. The following command demonstrates the syntax. We use the "—add-data" option twice and place two files into two folders. When the execution completes, we should find the spec file and the "build" and "dist" folders, as shown in Figure 4. We then can deploy the "dist\ MSSQLTips_GUI_app" folder to target machines.
pyinstaller --icon "MSSQLtips.ico" --windowed --add-data "C:\temp\MSSQLTips\myenv\Lib\site-packages\frozendict\VERSION;.\frozendict" --add-data "MSSQLtips.ico;." "MSSQLTips_GUI_app.py"
We copy the folder "dist\ MSSQLTips_GUI_app" to the "C:\Program Files (x86)\MSSQLTips" on a target machine without any development environment. Then, we create a shortcut and place the shortcut on the desktop. Figure 8 illustrates the shortcut icon. We can run the application by clicking the shortcut. The application should open a new window, as shown in Figure 9. Users can interact with this window.
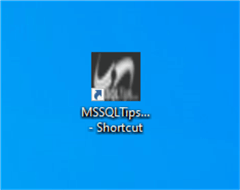
Figure 8 The Application Shortcut

Figure 9 The Python Application User Interface
5 – Making an Executable Using auto-py-to-exe
The auto-py-to-exe module uses a simple graphical interface and PyInstaller to perform the conversion. We continue to use the testing environment. We use the following command to install the module in the virtual environment:
pip install auto-py-to-exe
Next, we run the module by executing the following command line:
auto-py-to-exe
After running the command, we should see a pop-up window, as shown in Figure 10. If there is no Chrome on the machine, the auto-py-to-exe uses the default browser, and the interface may differ. We can config the interface according to the figure to create a deployment package. It is worth noting that the "Current Command" box shows the command line. When the user presses the "CONVERT .PY TO .EXE" button, auto-py-to-exe sends all the settings to PyInstaller, then receives the output from PyInstaller (Vollebregt, 2018).
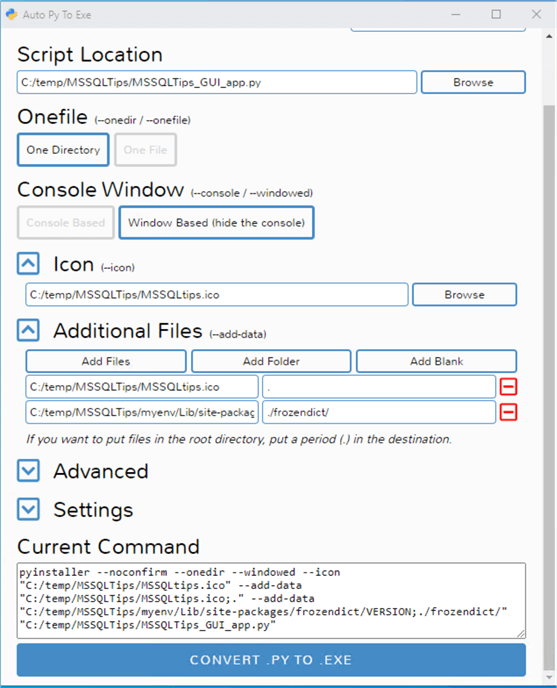
Figure 10 The Auto Py to Exe Interface
When the process completes, we find a line in the message output that says, "Moving project to: C:\temp\MSSQLTips\output." We then find the folder "MSSQLTips_GUI_app" in the "output" folder. We can use this folder to create a deployment package.
Summary
It isn't easy to distribute Python applications to end-users who are not developers. This tip introduced PyInstaller, which can create a one-folder bundle containing an executable. The executable can run independently of a python environment. We then made a deployment package from the folder. The deployment package simplifies the Python application deployment process. The article explored two ways to create an executable file. The first method used PyInstaller, and the second used auto-py-to-exe.
We started with setting up a testing environment. We then installed Pyinstaller in the virtual environment. Next, we briefly covered some Pyinstaller common command arguments. Running the command created a folder that contains an executable file and all requirements to run the executable. Next, we used the command arguments to add files to the folder. Finally, we tested this executable successfully on a target machine.
Next, we introduced auto-py-to-exe, which provides a friendly user interface to help quickly create executables. We provided a command to install the module and then ran the module and configured the interface. Once the conversion was completed, we saw the output folder in the current working folder. The output folder contains an executable and all the files and dependencies related to the executable file.
Reference
Cortesi, D. (2023). PyInstaller Manual. https://pyinstaller.org/en/stable/.
Jaworski, M. & Ziade, T. (2016). Expert Python Programming - Second Edition. Birmingham, UK: Packt Publishing.
Khandelwal, R. (2020). Easy Steps to Create an Executable in Python Using PyInstaller. https://medium.com/swlh/easy-steps-to-create-an-executable-in-python-using-pyinstaller-cc48393bcc64.
Lee, L. (2019). Using PyInstaller to Easily Distribute Python Applications. https://realpython.com/pyinstaller-python/.
Thapliyal, T. (2020). PyInstaller – Create Executable Python Files. https://www.askpython.com/python/pyinstaller-executable-files.
Vollebregt, B. (2018). Issues When Using auto-py-to-exe. https://nitratine.net/blog/post/issues-when-using-auto-py-to-exe/.
Next Steps
- The article covered the basics of using Pyinstaller to create an executable file. PyInstaller puts all the Python libraries required by a Python application into the dist folder. We can create a deployment package from the whole dist folder. Running the PyInstaller command makes a spec file containing specifications on creating executables from the Python application. Even though modifying the spec file is unnecessary, we should understand the spec file because the file allows us to add advanced settings (Khandelwal, 2020). In addition, other ways exist to freeze Python scripts to executable files, such as py2exe and cx_freeze. We can try other solutions when one method fails to generate the executable.
- Check out these related tips:
- Load Skype Meetings data via API into SQL Server using Python
- SQL Server Reporting with Python Flask
- Getting Started with Statistics using Python
- Load API Data to SQL Server Using Python and Generate Report with Power BI
- Load Data Asynchronously to SQL Server via an API and Python
- Twitter API to Execute Queries and Analyze Data in SQL Server
- Creating a Python Graphical User Interface with Tkinter
- Python Programming Tutorial with Top-Down Approach
About the author
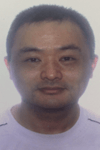
This author pledges the content of this article is based on professional experience and not AI generated.
View all my tips
Article Last Updated: 2023-03-29